2. 编写一个程序,该程序从Double类型的数字文件中获取输入,并将文件中数字的平均值输出到屏幕上。该文件只包含由空格和/或换行符分隔的双类型数字。用C++
时间: 2024-09-30 08:06:30 浏览: 7
为了编写这样一个C++程序,你可以使用`fstream`库来读取文件内容,然后遍历并计算所有数字的总和,最后除以数字的数量得到平均值。以下是简单的代码示例:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
double calculate_average(const std::string &file_path) {
double sum = 0;
int count = 0;
std::ifstream inputFile(file_path);
if (!inputFile.is_open()) {
std::cerr << "Failed to open file: " << file_path << std::endl;
return 0;
}
std::string line;
while (std::getline(inputFile, line)) {
std::istringstream iss(line);
double num;
if (iss >> num) {
sum += num;
count++;
} else {
std::cerr << "Invalid number found in the file." << std::endl;
}
}
inputFile.close();
if (count > 0) {
return sum / count;
} else {
return 0;
}
}
int main() {
const char* filePath = "numbers.txt"; // 替换为你实际的文件路径
double average = calculate_average(filePath);
if (average != 0) {
std::cout << "Average of numbers in the file is: " << average << std::endl;
}
return 0;
}
```
在这个程序中,我们首先打开文件,然后逐行读取。如果一行可以解析成一个double类型的数值,我们就累加到总和并增加计数。如果无法解析,则认为是无效的数据。最后,关闭文件并对总和进行平均运算。
注意:替换`"numbers.txt"`为你的实际文件路径。
相关推荐
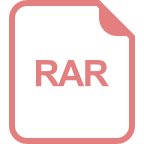
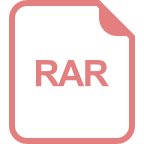














