字符串最长共有前缀
时间: 2023-07-25 10:16:27 浏览: 88
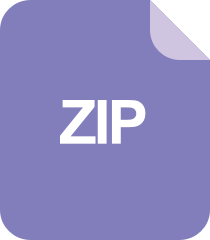
编写一个函数来查找字符串数组中的最长公共前缀
这是一个常见的字符串问题,可以使用 Trie 树(字典树)来解决。具体步骤如下:
1. 将所有字符串插入到 Trie 树中。
2. 从 Trie 树的根节点开始,遍历所有字符串的公共前缀,直到遇到第一个不同的字符或到达某个字符串的结尾。
3. 返回遍历过程中经过的所有字符,即为所有字符串的最长公共前缀。
代码示例(Python):
```python
class TrieNode:
def __init__(self):
self.children = {}
self.is_end = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for c in word:
if c not in node.children:
node.children[c] = TrieNode()
node = node.children[c]
node.is_end = True
def longest_common_prefix(self):
node = self.root
prefix = ""
while len(node.children) == 1 and not node.is_end:
c = list(node.children.keys())[0]
prefix += c
node = node.children[c]
return prefix
words = ["flower", "flow", "flight"]
trie = Trie()
for word in words:
trie.insert(word)
print(trie.longest_common_prefix()) # 输出 "fl"
```
注意事项:上述代码仅适用于字符串数组中至少有一个字符串的情况,如果字符串数组为空,需要特别处理。
阅读全文
相关推荐
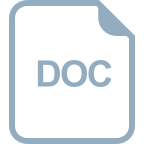
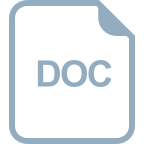


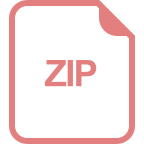
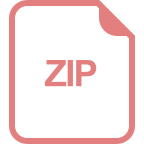
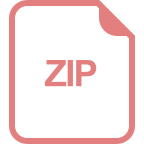
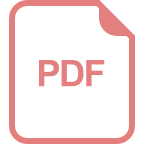
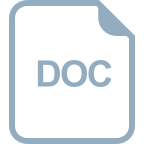
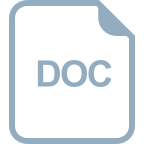
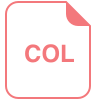




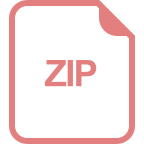
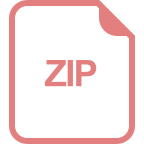
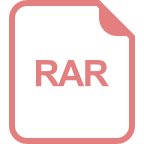