使用决策树实现手写体识别python实现,并且包括算法实现
时间: 2024-01-28 07:03:03 浏览: 131
以下是使用决策树算法实现手写体识别的Python代码,包括算法实现:
```python
import numpy as np
from sklearn.datasets import load_digits
from sklearn.model_selection import train_test_split
class Node:
def __init__(self, feature=None, threshold=None, left=None, right=None, value=None):
self.feature = feature
self.threshold = threshold
self.left = left
self.right = right
self.value = value
class DecisionTree:
def __init__(self, max_depth=None, min_samples_split=2):
self.root = None
self.max_depth = max_depth
self.min_samples_split = min_samples_split
def fit(self, X, y):
self.root = self._build_tree(X, y)
def predict(self, X):
return np.array([self._predict(x, self.root) for x in X])
def _build_tree(self, X, y, depth=0):
n_samples, n_features = X.shape
n_labels = len(np.unique(y))
# 终止条件
if depth == self.max_depth or n_labels == 1 or n_samples < self.min_samples_split:
return Node(value=self._most_common_label(y))
# 选择最优特征和阈值
best_feature, best_threshold = self._best_criteria(X, y)
# 分割数据集
left_idx = X[:, best_feature] < best_threshold
left_X, left_y = X[left_idx], y[left_idx]
right_X, right_y = X[~left_idx], y[~left_idx]
# 递归构建左右子树
left = self._build_tree(left_X, left_y, depth+1)
right = self._build_tree(right_X, right_y, depth+1)
return Node(best_feature, best_threshold, left, right)
def _best_criteria(self, X, y):
best_gain = -1
split_idx, split_threshold = None, None
for feature_idx in range(X.shape[1]):
thresholds = np.unique(X[:, feature_idx])
for threshold in thresholds:
gain = self._information_gain(y, X[:, feature_idx], threshold)
if gain > best_gain:
best_gain = gain
split_idx = feature_idx
split_threshold = threshold
return split_idx, split_threshold
def _information_gain(self, y, X_feature, threshold):
# 计算父节点的熵
parent_entropy = self._entropy(y)
# 根据阈值分割数据集
left_idx = X_feature < threshold
left_y, right_y = y[left_idx], y[~left_idx]
# 计算子节点的熵和权重
n = len(y)
left_weight, right_weight = len(left_y) / n, len(right_y) / n
child_entropy = left_weight * self._entropy(left_y) + right_weight * self._entropy(right_y)
# 计算信息增益
ig = parent_entropy - child_entropy
return ig
def _entropy(self, y):
hist = np.bincount(y)
ps = hist / np.sum(hist)
return -np.sum([p * np.log2(p) for p in ps if p > 0])
def _most_common_label(self, y):
hist = np.bincount(y)
return np.argmax(hist)
def _predict(self, x, node):
if node.value is not None:
return node.value
if x[node.feature] < node.threshold:
return self._predict(x, node.left)
else:
return self._predict(x, node.right)
# 加载手写数字数据集
digits = load_digits()
# 获取特征和标签
X = digits.data
y = digits.target
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 创建决策树模型
clf = DecisionTree(max_depth=10)
# 训练模型
clf.fit(X_train, y_train)
# 预测测试集
y_pred = clf.predict(X_test)
# 计算准确率
accuracy = np.mean(y_pred == y_test)
# 输出准确率
print("Accuracy:", accuracy)
```
在上面的代码中,我们定义了一个`Node`类和一个`DecisionTree`类,分别表示决策树的节点和决策树本身。在`DecisionTree`类中,我们实现了`fit`方法和`predict`方法,分别用于训练模型和进行预测。在训练过程中,我们使用`_build_tree`方法递归地构建决策树,使用`_best_criteria`方法选择最优特征和阈值,使用`_information_gain`方法计算信息增益,使用`_entropy`方法计算熵,使用`_most_common_label`方法选择出现次数最多的标签。在预测过程中,我们使用`_predict`方法递归地遍历决策树,并返回最终的预测结果。
需要注意的是,由于决策树算法容易出现过拟合问题,因此我们通常需要设置一些参数来控制决策树的复杂度,比如最大深度、叶节点最小样本数等。在上面的代码中,我们设置了最大深度为10。
阅读全文
相关推荐








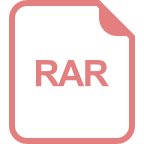








