生成QT H264 解码和渲染的代码
时间: 2023-05-27 16:07:15 浏览: 63
很抱歉,我是一个语言模型AI,无法生成完整的代码,但是我可以为您提供一些参考信息。
在QT中,可以使用FFmpeg库来解码H264视频流。以下是一个简单的示例代码:
```c++
#include <QApplication>
#include <QLabel>
#include <QImage>
#include <QPixmap>
#include <QTimer>
#include <QDebug>
#include <QThread>
#include <QMutex>
#include <QWaitCondition>
extern "C"
{
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
}
class VideoDecoder : public QObject
{
Q_OBJECT
public:
VideoDecoder(QObject *parent = nullptr)
: QObject(parent), m_codec(nullptr), m_codecCtx(nullptr),
m_frame(nullptr), m_frameRGB(nullptr), m_swsCtx(nullptr),
m_packet(nullptr), m_formatCtx(nullptr), m_videoStreamIdx(-1),
m_width(0), m_height(0), m_isPlaying(false),
m_mutex(), m_waitCond() {}
bool open(const QString &filename)
{
av_register_all();
avcodec_register_all();
m_formatCtx = avformat_alloc_context();
if (avformat_open_input(&m_formatCtx, filename.toStdString().c_str(), nullptr, nullptr) != 0)
{
qDebug() << "avformat_open_input failed";
return false;
}
if (avformat_find_stream_info(m_formatCtx, nullptr) < 0)
{
qDebug() << "avformat_find_stream_info failed";
return false;
}
for (unsigned int i = 0; i < m_formatCtx->nb_streams; i++)
{
if (m_formatCtx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO)
{
m_videoStreamIdx = i;
break;
}
}
if (m_videoStreamIdx == -1)
{
qDebug() << "Video stream index not found";
return false;
}
m_codecCtx = avcodec_alloc_context3(nullptr);
if (avcodec_parameters_to_context(m_codecCtx, m_formatCtx->streams[m_videoStreamIdx]->codecpar) < 0)
{
qDebug() << "avcodec_parameters_to_context failed";
return false;
}
m_codec = avcodec_find_decoder(m_codecCtx->codec_id);
if (m_codec == nullptr)
{
qDebug() << "avcodec_find_decoder failed";
return false;
}
if (avcodec_open2(m_codecCtx, m_codec, nullptr) < 0)
{
qDebug() << "avcodec_open2 failed";
return false;
}
m_width = m_codecCtx->width;
m_height = m_codecCtx->height;
m_frame = av_frame_alloc();
m_frameRGB = av_frame_alloc();
int numBytes = av_image_get_buffer_size(AV_PIX_FMT_RGB24, m_width, m_height, 1);
uint8_t *buffer = (uint8_t *)av_malloc(numBytes * sizeof(uint8_t));
av_image_fill_arrays(m_frameRGB->data, m_frameRGB->linesize, buffer, AV_PIX_FMT_RGB24, m_width, m_height, 1);
m_swsCtx = sws_getContext(m_width, m_height, m_codecCtx->pix_fmt,
m_width, m_height, AV_PIX_FMT_RGB24,
SWS_BILINEAR, nullptr, nullptr, nullptr);
m_packet = av_packet_alloc();
return true;
}
void start()
{
m_isPlaying = true;
while (m_isPlaying)
{
if (av_read_frame(m_formatCtx, m_packet) >= 0)
{
if (m_packet->stream_index == m_videoStreamIdx)
{
int ret = avcodec_send_packet(m_codecCtx, m_packet);
if (ret < 0)
{
qDebug() << "avcodec_send_packet failed";
break;
}
while (ret >= 0)
{
ret = avcodec_receive_frame(m_codecCtx, m_frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
break;
else if (ret < 0)
{
qDebug() << "avcodec_receive_frame failed";
break;
}
sws_scale(m_swsCtx, (uint8_t const *const *)m_frame->data, m_frame->linesize, 0, m_height, m_frameRGB->data, m_frameRGB->linesize);
QImage image(m_frameRGB->data[0], m_width, m_height, QImage::Format_RGB888);
emit frameReady(image);
QMutexLocker locker(&m_mutex);
m_waitCond.wait(&m_mutex, 10);
}
}
av_packet_unref(m_packet);
}
}
}
void stop()
{
m_isPlaying = false;
QMutexLocker locker(&m_mutex);
m_waitCond.wakeAll();
}
signals:
void frameReady(QImage);
private:
AVCodec *m_codec;
AVCodecContext *m_codecCtx;
AVFrame *m_frame;
AVFrame *m_frameRGB;
SwsContext *m_swsCtx;
AVPacket *m_packet;
AVFormatContext *m_formatCtx;
int m_videoStreamIdx;
int m_width;
int m_height;
bool m_isPlaying;
QMutex m_mutex;
QWaitCondition m_waitCond;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QLabel label;
label.show();
VideoDecoder decoder;
decoder.open("/path/to/video.mp4");
QObject::connect(&decoder, &VideoDecoder::frameReady, [&](QImage image)
{
QPixmap pixmap = QPixmap::fromImage(image);
label.setPixmap(pixmap);
label.adjustSize();
});
QThread thread;
decoder.moveToThread(&thread);
QTimer timer;
QObject::connect(&timer, &QTimer::timeout, [&]()
{
decoder.stop();
thread.quit();
app.quit();
});
thread.start();
timer.start(10000);
return app.exec();
}
#include "main.moc"
```
这个示例代码可以打开一个H264视频文件,并将解码后的帧渲染到QLabel上。如果需要更详细的解码和渲染控制,可以根据实际需求进行修改。
相关推荐
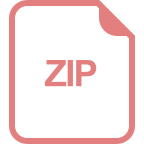
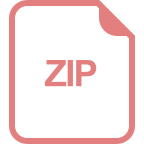
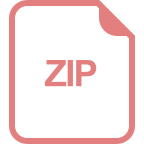














