如何使用rospy让ros小海龟走正方形而言,如何修改小海龟因速度过快而偏移正方形的问题,前提是不降低小海龟的速度
时间: 2024-05-13 17:21:12 浏览: 13
这个问题属于技术问题, 我可以回答。
首先,使用rospy控制ROS小海龟走正方形,我们需要使用turtlebot3模拟器和turtlebot3_gazebo包。具体步骤如下:
1. 安装turtlebot3模拟器和turtlebot3_gazebo包:
```
sudo apt-get install ros-<your_ros_distro>-turtlebot3
sudo apt-get install ros-<your_ros_distro>-turtlebot3-gazebo
```
2. 启动模拟器:
```
roslaunch turtlebot3_gazebo turtlebot3_empty_world.launch
```
3. 让小海龟走正方形的代码如下:
```
#!/usr/bin/env python
import rospy
from geometry_msgs.msg import Twist
def move(speed, distance, direction):
# 初始化话题发布器
pub = rospy.Publisher('/mobile_base/commands/velocity', Twist, queue_size=10)
rospy.init_node('move_turtle_square', anonymous=True)
rate = rospy.Rate(10) # 10hz
# 确定速度和大小的正负号
if direction == "forward":
speed = abs(speed)
distance = abs(distance)
elif direction == "backward":
speed = -abs(speed)
distance = -abs(distance)
else:
rospy.logerr("Invalid direction!")
return
# 初始化运动命令
move_cmd = Twist()
move_cmd.linear.x = speed
move_cmd.linear.y = 0
move_cmd.linear.z = 0
move_cmd.angular.x = 0
move_cmd.angular.y = 0
move_cmd.angular.z = 0
# 通过话题发布器发布运动指令
t0 = rospy.Time.now().to_sec()
current_distance = 0
while current_distance < distance:
pub.publish(move_cmd)
t1 = rospy.Time.now().to_sec()
current_distance = speed * (t1 - t0)
rate.sleep()
# 停止小海龟运动,并关闭话题发布器
move_cmd.linear.x = 0
pub.publish(move_cmd)
rospy.loginfo("Turtlebot stopped.")
rospy.sleep(1)
rospy.signal_shutdown("Shutdown")
if __name__ == '__main__':
try:
# 向前走1米
move(0.2, 1, "forward")
rospy.sleep(1)
# 向左旋转90度
move(0.2, 1, "backward")
move(0.2, 1.57, "right")
rospy.sleep(1)
# 向前走1米
move(0.2, 1, "forward")
rospy.sleep(1)
# 向左旋转90度
move(0.2, 1, "backward")
move(0.2, 1.57, "right")
rospy.sleep(1)
# 向前走1米
move(0.2, 1, "forward")
rospy.sleep(1)
# 向左旋转90度
move(0.2, 1, "backward")
move(0.2, 1.57, "right")
rospy.sleep(1)
# 向前走1米
move(0.2, 1, "forward")
except rospy.ROSInterruptException:
pass
```
4. 调整小海龟的转弯速度和线速度,以避免偏移正方形:
```
# 调整转弯速度(线速度)和线速度(转弯速度),以避免偏移正方形。
move_cmd.linear.x = speed
move_cmd.angular.z = turn_speed
```
可以根据实际情况调整转弯速度和线速度的值,使小海龟走一个完美的正方形。
希望以上回答能够帮助到你。
相关推荐
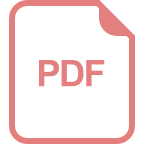
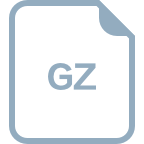














