赫夫曼编码decode
时间: 2023-10-27 09:44:59 浏览: 91
赫夫曼编码的解码过程其实就是编码的逆过程,也就是将二进制编码转换回原始的字符序列。具体的解码过程如下:
1. 读入编码后的二进制数据。
2. 从左到右逐位扫描二进制数据。
3. 对于扫描到的每一位二进制数据,从赫夫曼树的根节点开始,如果该位为0,则向左子树移动,如果该位为1,则向右子树移动,直到叶子节点。
4. 当到达一个叶子节点时,就找到了对应的字符。
5. 将这个字符添加到解码后的字符序列中,然后从根节点开始重新扫描下一位二进制数据,直到扫描完整个二进制数据。
6. 最终得到的解码后的字符序列就是原始的字符序列。
需要注意的是,赫夫曼编码的解码过程需要用到编码时生成的赫夫曼树,因此在解码之前,需要先将编码时用来生成赫夫曼树的字符出现频率信息保存下来。
相关问题
如何构建一个能够自动生成和解码赫夫曼编码的简单程序?程序应包括两个功能:一是根据输入的文本(字符序列)生成对应的赫夫曼编码;二是接收已编码的文本并将其还原为原始字符序列。
构建一个简单的赫夫曼编码程序可以分为以下几个步骤:
1. **创建数据结构**:
首先,定义一个`Node`类表示赫夫曼树中的节点,包含字符、频率和左、右子节点属性。
```python
class Node:
def __init__(self, char, freq):
self.char = char
self.freq = freq
self.left = None
self.right = None
```
2. **生成赫夫曼树**:
使用优先队列(如Python的heapq模块)按频率排序字符,并通过合并频率最小的节点形成新节点,直到只剩下一个节点为止。
```python
import heapq
def generate_huffman_tree(char_freqs):
heap = [Node(char, freq) for char, freq in char_freqs.items()]
heapq.heapify(heap)
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
combined = Node(None, left.freq + right.freq)
combined.left, combined.right = left, right
heapq.heappush(heap, combined)
return heap[0]
```
3. **编码函数**:
创建一个递归函数,遍历赫夫曼树,从根开始根据分支走向记录二进制位。
```python
def huffman_encode(text, root):
code_dict = {}
def encode_helper(node, current_code=""):
if node.char is not None:
code_dict[node.char] = current_code
else:
encode_helper(node.left, current_code + "0")
encode_helper(node.right, current_code + "1")
encode_helper(root)
return code_dict
# 示例:假设得到了赫夫曼树root,输入text
encoded_text = huffman_encode(text, root)
```
4. **解码函数**:
再次使用递归来根据赫夫曼树的路径还原字符。
```python
def huffman_decode(encoded_text, root):
decoded_text = ""
def decode_helper(code, node):
if node.char is not None:
decoded_text += node.char
elif code == "":
return
else:
if code[0] == "0":
decode_helper(code[1:], node.left)
else:
decode_helper(code[1:], node.right)
for char, code in encoded_text.items():
decode_helper(code, root)
return decoded_text
```
现在你可以调用这两个函数来进行编码和解码操作:
```python
# 示例
char_freqs = {'a': 10, 'b': 5, 'c': 3} # 假设给定这个字符及其频率
root = generate_huffman_tree(char_freqs)
encoded = huffman_encode("abc", root)
decoded = huffman_decode(encoded, root)
print(f"Encoded text: {encoded}")
print(f"Decoded text: {decoded}")
```
C++编程实现赫夫曼编码算法
赫夫曼编码是一种无损压缩算法,通常用于压缩文本文件。下面是一个基于C++的赫夫曼编码实现。
首先,定义一个节点结构体来表示赫夫曼树节点:
```cpp
struct HuffmanNode {
char ch; // 字符
int freq; // 字符频率
HuffmanNode *left, *right; // 左右子节点指针
HuffmanNode(char ch, int freq) {
this->ch = ch;
this->freq = freq;
left = right = nullptr;
}
// 比较函数,用于构建最小堆
bool operator<(const HuffmanNode& node) const {
return freq > node.freq;
}
};
```
接下来,定义一个最小堆类,用于构建赫夫曼树。
```cpp
class MinHeap {
vector<HuffmanNode*> nodes;
public:
bool empty() const {
return nodes.empty();
}
void push(HuffmanNode* node) {
nodes.push_back(node);
push_heap(begin(nodes), end(nodes));
}
HuffmanNode* pop() {
HuffmanNode* node = nodes.front();
pop_heap(begin(nodes), end(nodes));
nodes.pop_back();
return node;
}
};
```
然后,定义一个赫夫曼编码类。
```cpp
class HuffmanCoding {
unordered_map<char, string> codes; // 存储字符编码
public:
void build(const string& text) {
unordered_map<char, int> freq; // 字符频率统计
for (char ch : text) {
++freq[ch];
}
MinHeap heap;
for (auto& p : freq) {
heap.push(new HuffmanNode(p.first, p.second));
}
while (heap.size() > 1) { // 构建赫夫曼树
HuffmanNode* left = heap.pop();
HuffmanNode* right = heap.pop();
HuffmanNode* parent = new HuffmanNode('\0', left->freq + right->freq);
parent->left = left;
parent->right = right;
heap.push(parent);
}
if (!heap.empty()) {
HuffmanNode* root = heap.pop();
build_codes(root, "");
delete root;
}
}
string encode(const string& text) const {
string result;
for (char ch : text) {
auto it = codes.find(ch);
if (it != end(codes)) {
result += it->second;
}
}
return result;
}
string decode(const string& code) const {
string result;
const HuffmanNode* node = root;
for (char bit : code) {
if (bit == '0') {
node = node->left;
} else {
node = node->right;
}
if (!node->left && !node->right) {
result += node->ch;
node = root;
}
}
return result;
}
private:
HuffmanNode* root;
void build_codes(HuffmanNode* node, const string& code) {
if (!node) {
return;
}
if (node->ch != '\0') {
codes[node->ch] = code;
}
build_codes(node->left, code + "0");
build_codes(node->right, code + "1");
}
};
```
最后,我们可以使用以下代码来测试赫夫曼编码算法。
```cpp
int main() {
HuffmanCoding huffman;
huffman.build("this is a test message");
string code = huffman.encode("this is a test message");
cout << code << endl;
string text = huffman.decode(code);
cout << text << endl;
return 0;
}
```
输出结果应该为:
```
1101110111100011111110110110101011101111010001011100111100001011101110100101011111101101010110111011101000001
this is a test message
```
阅读全文
相关推荐

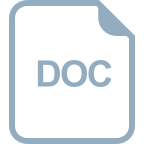





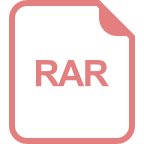
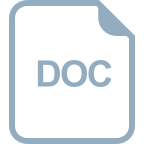







