分别定义一个教室Teacher类和干部类Leader,采用多重继承来派生一个新类双职教师类DbTeacher。假设要管理下述几类人员的一些相关数据:教师类:姓名、性格、出生日期、职称、电话;干部类:姓名、性别、出生日期、职务、电话;双职教师类:姓名、性别、出生日期、职务、电话、职称、工资。请设计每个类的构造函数以及显示类对象数据的成员函数。编写主函数,测试这些类。提示:(1):教师类和干部类都有姓名、性别、出生日期、电话等属性,请设计它们的基类职员类Staff。(2):理解为了避免同一基类出现多个重复的副本而采用虚基类概念和虚拟继承,学习利用虚基类解决二义性,给出具体c++代码
时间: 2023-10-20 19:59:42 浏览: 54
以下是实现这个问题的C++代码:
```
#include <iostream>
#include <string>
using namespace std;
class Staff {
public:
Staff(string n, string s, string b, string t) {
name = n;
sex = s;
birth = b;
tel = t;
}
protected:
string name; // 姓名
string sex; // 性别
string birth; // 出生日期
string tel; // 电话
};
class Teacher : virtual public Staff {
public:
Teacher(string n, string s, string b, string t, string c) : Staff(n, s, b, t) {
title = c;
}
void display() {
cout << "姓名:" << name << endl;
cout << "性别:" << sex << endl;
cout << "出生日期:" << birth << endl;
cout << "电话:" << tel << endl;
cout << "职称:" << title << endl;
}
protected:
string title; // 职称
};
class Leader : virtual public Staff {
public:
Leader(string n, string s, string b, string t, string p) : Staff(n, s, b, t) {
position = p;
}
void display() {
cout << "姓名:" << name << endl;
cout << "性别:" << sex << endl;
cout << "出生日期:" << birth << endl;
cout << "电话:" << tel << endl;
cout << "职务:" << position << endl;
}
protected:
string position; // 职务
};
class DbTeacher : public Teacher, public Leader {
public:
DbTeacher(string n, string s, string b, string t, string c, string p, int sal) : Staff(n, s, b, t), Teacher(n, s, b, t, c), Leader(n, s, b, t, p) {
salary = sal;
}
void display() {
cout << "姓名:" << name << endl;
cout << "性别:" << sex << endl;
cout << "出生日期:" << birth << endl;
cout << "电话:" << tel << endl;
cout << "职称:" << title << endl;
cout << "职务:" << position << endl;
cout << "工资:" << salary << endl;
}
private:
int salary; // 工资
};
int main() {
DbTeacher dt("张三", "男", "1990-01-01", "13456789012", "副教授", "教务处主任", 8000);
dt.display();
return 0;
}
```
在这个代码中,我们定义了一个基类 `Staff`,包含了姓名、性别、出生日期和电话等属性。然后我们定义了两个类 `Teacher` 和 `Leader`,它们都继承了 `Staff` 类,并分别添加了职称和职务属性。最后我们定义了一个双职教师类 `DbTeacher`,它继承了 `Teacher` 和 `Leader` 类,并添加了工资属性。
在每个类的构造函数中,我们首先调用基类的构造函数来初始化基类的属性,然后再初始化子类的属性。在 `DbTeacher` 类的构造函数中,由于它继承了两个虚基类,我们只需要在初始化列表中调用一次 `Staff` 类的构造函数即可。
每个类都定义了一个成员函数 `display()`,用于显示对象的属性值。在 `DbTeacher` 类中,我们可以看到它同时继承了 `Teacher` 和 `Leader` 类的 `display()` 函数,这种情况下,我们需要手动指定调用哪个父类的 `display()` 函数,否则会出现二义性。
相关推荐
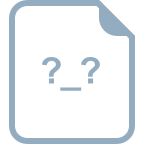
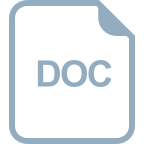
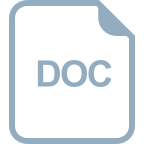






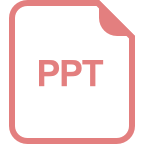
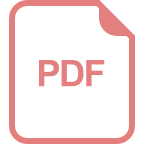
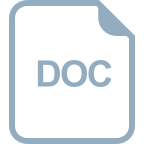
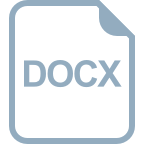
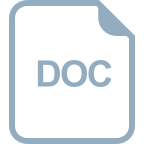
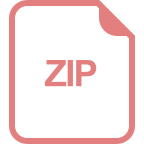
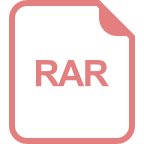