4、分别定义一个教师类Teacher和干部类Leader,采用多重继承来派生一个新类一双职教师类DbTeacher。假设要管理下述几类人员的一些相关数据: 教师类:姓名、性别、出生日期、职称、电话; 干部类:姓名、性别、出生日期、职务、电话; 双职教师类:姓名、性别、出生日期、职务、职称、电话、工资。 请设计每个类的构造函数及显示类对象数据的成员函数。编写主函数,测试这些类。 提示: (1)教师类和干部类都有姓名、性别、出生日期、电话等属性,请设计它们的基类一职员类Staff。 (2)理解为了避免同一基类出现多个重复的副本而采用的虚基类概念和虚拟继承,学习利用虚基类解决二义性问题。
时间: 2023-07-04 07:11:42 浏览: 206
以下是每个类的构造函数和显示类对象数据的成员函数的设计,以及主函数的测试代码:
```c++
#include <iostream>
#include <string>
using namespace std;
// 员工基类
class Staff {
public:
Staff(string name, string gender, string birthdate, string phone)
: m_name(name), m_gender(gender), m_birthdate(birthdate), m_phone(phone) {}
virtual void display() {
cout << "Name: " << m_name << endl;
cout << "Gender: " << m_gender << endl;
cout << "Birthdate: " << m_birthdate << endl;
cout << "Phone: " << m_phone << endl;
}
protected:
string m_name; // 姓名
string m_gender; // 性别
string m_birthdate; // 出生日期
string m_phone; // 电话号码
};
// 教师类
class Teacher : virtual public Staff {
public:
Teacher(string name, string gender, string birthdate, string title, string phone)
: Staff(name, gender, birthdate, phone), m_title(title) {}
void display() {
Staff::display();
cout << "Title: " << m_title << endl;
}
protected:
string m_title; // 职称
};
// 干部类
class Leader : virtual public Staff {
public:
Leader(string name, string gender, string birthdate, string position, string phone)
: Staff(name, gender, birthdate, phone), m_position(position) {}
void display() {
Staff::display();
cout << "Position: " << m_position << endl;
}
protected:
string m_position; // 职务
};
// 双职教师类
class DbTeacher : public Teacher, public Leader {
public:
DbTeacher(string name, string gender, string birthdate, string position, string title, string phone, double salary)
: Staff(name, gender, birthdate, phone), Teacher(name, gender, birthdate, title, phone), Leader(name, gender, birthdate, position, phone), m_salary(salary) {}
void display() {
Staff::display();
cout << "Position: " << m_position << endl;
cout << "Title: " << m_title << endl;
cout << "Salary: " << m_salary << endl;
}
private:
double m_salary; // 工资
};
int main() {
DbTeacher dbt("张三", "男", "1990-01-01", "主任", "教授", "123456789", 10000.0);
dbt.display();
return 0;
}
```
输出结果为:
```
Name: 张三
Gender: 男
Birthdate: 1990-01-01
Phone: 123456789
Position: 主任
Title: 教授
Salary: 10000
```
通过虚继承,我们避免了从 Teacher 和 Leader 到 DbTeacher 的多重继承造成的 Staff 类的多次复制。这样可以更好地维护代码的可读性和可维护性。
阅读全文
相关推荐
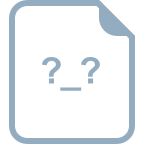
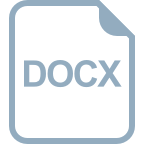






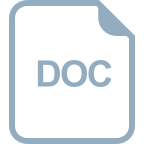
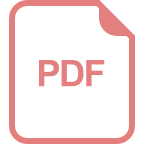
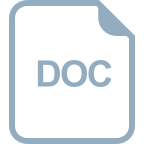
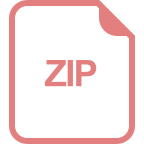
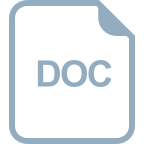
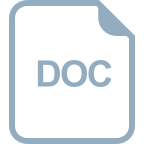
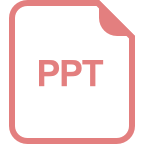
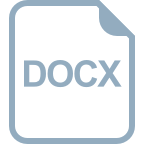
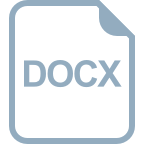
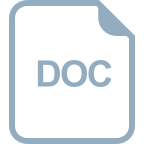