分别定义一个教师类Teacher和干部类Leader,采用多重继承派生一个新类一双职教师类。假设要管理下述几类人员的一些相关数据: 教师类:姓名、性别、出生日期、职称、电话; 干部类:姓名、性别、出生日期、职务、电话; 双职教师类:姓名、性别、出生日期、职务、职称、电话、工资。 请设计每个类的构造函数及显示类对象数据的成员函数。编写主函数,测试这些类。
时间: 2023-07-04 15:11:16 浏览: 128
下面是每个类的定义及相关函数的实现:
```c++
#include<iostream>
using namespace std;
// 教师类
class Teacher {
protected:
string name; // 姓名
string gender; // 性别
string birthday; // 出生日期
string title; // 职称
string phone; // 电话
public:
// 构造函数
Teacher(string n, string g, string b, string t, string p) {
name = n;
gender = g;
birthday = b;
title = t;
phone = p;
}
// 显示教师类对象数据的成员函数
void display() {
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "出生日期:" << birthday << endl;
cout << "职称:" << title << endl;
cout << "电话:" << phone << endl;
}
};
// 干部类
class Leader {
protected:
string name; // 姓名
string gender; // 性别
string birthday; // 出生日期
string position; // 职务
string phone; // 电话
public:
// 构造函数
Leader(string n, string g, string b, string p, string ph) {
name = n;
gender = g;
birthday = b;
position = p;
phone = ph;
}
// 显示干部类对象数据的成员函数
void display() {
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "出生日期:" << birthday << endl;
cout << "职务:" << position << endl;
cout << "电话:" << phone << endl;
}
};
// 双职教师类
class DoubleTeacher : public Teacher, public Leader {
protected:
string salary; // 工资
public:
// 构造函数
DoubleTeacher(string n, string g, string b, string t, string p, string ph, string s)
: Teacher(n, g, b, t, ph), Leader(n, g, b, p, ph), salary(s) {}
// 显示双职教师类对象数据的成员函数
void display() {
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "出生日期:" << birthday << endl;
cout << "职务:" << position << endl;
cout << "职称:" << title << endl;
cout << "电话:" << phone << endl;
cout << "工资:" << salary << endl;
}
};
int main() {
DoubleTeacher dt("张三", "男", "1990-01-01", "高级讲师", "系主任", "123456789", "8000");
dt.display();
return 0;
}
```
这段代码定义了三个类:`Teacher`、`Leader`、`DoubleTeacher`。其中,`Teacher`类和`Leader`类都有自己的构造函数和显示对象数据的成员函数,`DoubleTeacher`类继承了`Teacher`类和`Leader`类,并且在自己的构造函数中调用了基类的构造函数。最后,在主函数中,我们创建了一个`DoubleTeacher`类的对象`dt`,并调用了它的`display()`函数来显示对象的数据。
阅读全文
相关推荐
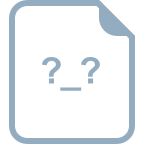






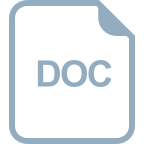
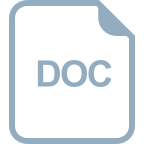
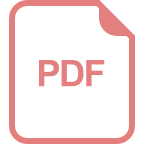
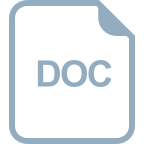
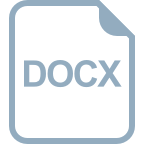
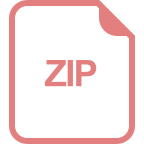
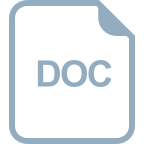
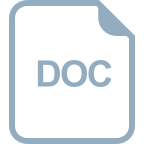
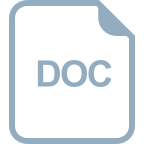
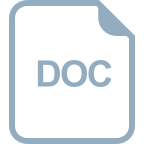
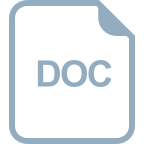