在vue中使用addEventListener添加鼠标移动事件,在鼠标在鼠标mouseup时清除移动事件,且能拿到移动后的event
时间: 2023-11-28 14:48:55 浏览: 164
可以尝试以下代码:
```
<template>
<div ref="box" @mousedown="handleMouseDown" @mouseup="handleMouseUp"></div>
</template>
<script>
export default {
methods: {
handleMouseDown() {
this.$refs.box.addEventListener('mousemove', this.handleMouseMove)
},
handleMouseMove(event) {
// 在移动时做一些操作
},
handleMouseUp(event) {
this.$refs.box.removeEventListener('mousemove', this.handleMouseMove)
// 在鼠标抬起时做一些操作,event也可以拿到
}
}
}
</script>
```
在鼠标按下时添加鼠标移动事件,在鼠标抬起时清除鼠标移动事件,并且可以拿到移动后的event对象。注意要使用`removeEventListener`来清除事件监听,否则会导致内存泄漏。
相关问题
如何防止Vue中的方法在鼠标快速滑动时意外触发?
在Vue中,为了防止由于用户快速连续操作导致方法无意中频繁触发,可以采用以下几种策略:
1. **事件修饰符**:Vue的`v-on`指令支持一些修饰符来控制事件处理。例如,`@touchstart.prevent`会阻止元素的默认触摸行为并阻止冒泡,这有助于防止因快速滑动而多次触发。
```html
<template>
<div @touchstart.prevent="handleSwipe">
<!-- ... -->
</div>
</template>
<script>
export default {
methods: {
handleSwipe(event) {
// 处理滑动事件的逻辑
}
}
}
</script>
```
2. **防抖(debounce)和节流(throttle)**:在需要频繁响应用户的输入时,可以使用JavaScript库如lodash的`_.debounce`或`_.throttle`函数来限制方法的执行频率,比如只在用户停止滑动一段时间后才执行回调。
```javascript
import { debounce } from 'lodash';
methods: {
handleSwipe = debounce(this.handleSwipeLogic, 300) // 指定延迟时间
}
// 使用
handleSwipe()
```
3. **使用自定义指令**:如果某个特定事件需要特殊处理,可以在组件上创建一个自定义指令,通过设置适当的条件来避免快速触发。
```html
<template>
<button v-stop-swipe="handleSwipe">点击</button>
</template>
<script>
export default {
directives: {
stopSwipe(el, binding, vnode) {
const handler = binding.value;
let timeoutId;
el.addEventListener('mousedown', start);
el.addEventListener('mouseup', end);
function start() {
timeoutId = setTimeout(() => {
handler();
}, 500); // 可调整为更长的时间间隔
}
function end() {
clearTimeout(timeoutId);
}
}
},
methods: {
handleSwipe() {
// ...
}
}
}
</script>
```
<template> <div class="container"> <div class="button" ref="buttonRef" :style="buttonStyle" @mousedown="startDrag" @touchstart="startDrag" > Button </div> </div> </template> <script> export default { data() { return { buttonIndex: 0, isDragging: false, initialPosition: { x: 0, y: 0 }, currentPosition: { x: 0, y: 0 } }; }, computed: { buttonStyle() { return { transform: `translate(${this.currentPosition.x}px, ${this.currentPosition.y}px)` }; } }, methods: { moveButton() { this.buttonIndex = Math.floor(Math.random() * 24); }, startDrag(event) { event.preventDefault(); // 计算初始位置 const boundingRect = this.$refs.buttonRef.getBoundingClientRect(); this.initialPosition = { x: event.clientX || event.touches[0].clientX, y: event.clientY || event.touches[0].clientY }; // 更新当前位置 this.currentPosition = { x: boundingRect.left, y: boundingRect.top }; // 监听移动和释放事件 window.addEventListener('mousemove', this.handleDrag); window.addEventListener('touchmove', this.handleDrag, { passive: false }); window.addEventListener('mouseup', this.stopDrag); window.addEventListener('touchend', this.stopDrag); }, handleDrag(event) { event.preventDefault(); // 计算移动距离 const clientX = event.clientX || event.touches[0].clientX; const clientY = event.clientY || event.touches[0].clientY; const deltaX = clientX - this.initialPosition.x; const deltaY = clientY - this.initialPosition.y; // 更新当前位置 this.currentPosition = { x: this.currentPosition.x + deltaX, y: this.currentPosition.y + deltaY }; }, stopDrag() { // 停止拖动,移除事件监听器 window.removeEventListener('mousemove', this.handleDrag); window.removeEventListener('touchmove', this.handleDrag); window.removeEventListener('mouseup', this.stopDrag); window.removeEventListener('touchend', this.stopDrag); } } }; </script> <style> .container { display: flex; align-items: center; justify-content: center; height: 100vh; } .button { width: 80px; height: 40px; background-color: #ccc; display: flex; align-items: center; justify-content: center; cursor: pointer; position: absolute; } </style> 执行时出现了以下错误[Vue warn]: Error in v-on handler: "TypeError: this.$refs.buttonRef.getBoundingClientRect is not a function" (found at pages/Dragging/Dragging.vue:1) 22:21:10.359 TypeError: this.$refs.buttonRef.getBoundingClientRect is not a function怎么改正
这个错误是因为`getBoundingClientRect()`方法不适用于Vue组件的ref属性。要解决这个问题,你可以使用`$el`来访问DOM元素并调用`getBoundingClientRect()`方法。修改代码如下:
```javascript
startDrag(event) {
event.preventDefault();
// 计算初始位置
const boundingRect = this.$refs.buttonRef.$el.getBoundingClientRect();
// 其他代码...
},
```
通过`this.$refs.buttonRef.$el`可以获取到引用的DOM元素,然后再调用`getBoundingClientRect()`方法即可。这样就能正确获取按钮元素的位置信息了。记得在其他地方也要相应地修改代码。希望对你有帮助!
阅读全文
相关推荐
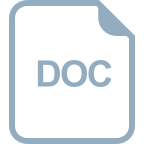
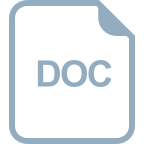
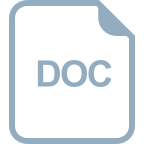
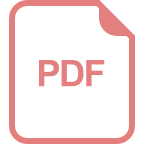
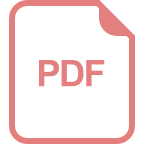











