C++有向邻接矩阵找到最深路径,写一个举例函数
时间: 2024-12-25 15:27:36 浏览: 16
在C++中,要实现有向邻接矩阵表示的图中找到从某个起点到终点的最深路径,可以使用深度优先搜索(Depth First Search, DFS)算法。下面是一个简单的DFS函数,它会返回从给定节点到最远可达节点的距离:
```cpp
#include <iostream>
using namespace std;
// 定义邻接矩阵边的数据结构
typedef struct {
int destination; // 目标节点
int weight; // 权重(假设无权重时为1)
} AdjacentEdge;
// 用于存储深度信息的辅助结构体
struct NodeInfo {
int depth;
};
// 深度优先搜索函数
int dfs(MGraph graph, int start, int end, vector<NodeInfo>& visited) {
visited[start].depth = 0;
queue<int> q;
q.push(start);
while (!q.empty()) {
int current = q.front();
q.pop();
if (current == end) {
return visited[end].depth; // 找到了目标节点,返回其深度
}
for (int i = 0; i < graph.n; ++i) {
if (graph.arc[current][i] != 0 && visited[i].depth == -1) {
visited[i].depth = visited[current].depth + 1;
q.push(i);
}
}
}
return -1; // 如果未找到路径,返回-1表示无路可达
}
// 示例调用
int main() {
MGraph g;
// 假设g.arc已经初始化好了邻接矩阵
int start = 0, end = 4; // 起点和终点
vector<NodeInfo> nodeVis(maxSize, {-1}); // 初始化访问标记
int result = dfs(g, start, end, nodeVis);
if (result != -1) {
cout << "The deepest path from node " << start << " to node " << end << " has a depth of " << result << endl;
} else {
cout << "No path exists between the nodes." << endl;
}
return 0;
}
```
在这个例子中,`dfs`函数首先将起始节点的深度设置为0,然后使用广度优先队列(`queue`)来遍历图。如果遇到目标节点,返回其深度;若未找到,则返回-1表示无路径。
阅读全文
相关推荐





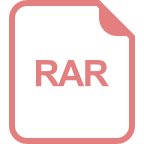

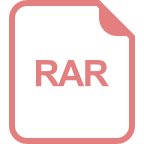
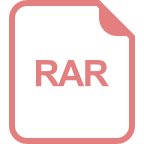
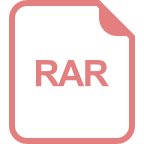
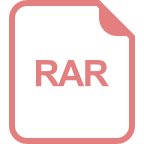
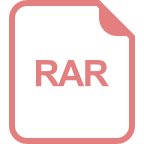

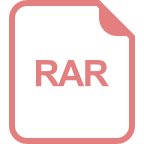




