数据结构课程设计c++图书管理系统
时间: 2025-01-04 09:30:22 浏览: 9
### 关于C++图书管理系统的数据结构课程设计
#### 设计概述
为了构建一个高效的图书管理系统,采用适当的数据结构和控制结构至关重要。该系统旨在管理图书馆中的书籍信息、用户权限、借阅历史等核心功能[^1]。
#### 主要模块说明
- **书籍信息管理**:负责处理书籍的增删改查操作;
- **用户权限管理**:定义不同用户的权限级别及其对应的操作范围;
- **借阅历史记录**:追踪每本书籍的借还情况;
- **图形用户界面**:提供友好的交互环境给最终使用者;
- **数据安全保障机制**:确保所有敏感信息的安全存储与传输。
#### 示例代码实现方案
##### 头文件 `Book.h`
```cpp
#ifndef BOOK_H_
#define BOOK_H_
#include <string>
class Book {
private:
std::string title;
std::string author;
int id; // Unique identifier for each book
public:
Book(std::string t, std::string a, int i);
void setTitle(const std::string& newTitle);
const std::string& getTitle() const;
void setAuthor(const std::string& newAuthor);
const std::string& getAuthor() const;
void setId(int newId);
int getId() const;
};
#endif /*BOOK_H_*/
```
##### 源文件 `Book.cpp`
```cpp
#include "Book.h"
// Constructor implementation
Book::Book(std::string t, std::string a, int i):title(t),author(a),id(i){}
void Book::setTitle(const std::string &newTitle){
this->title=newTitle;
}
const std::string &Book::getTitle() const{
return this->title;
}
void Book::setAuthor(const std::string &newAuthor){
this->author=newAuthor;
}
const std::string &Book::getAuthor() const{
return this->author;
}
void Book::setId(int newId){
this->id=newId;
}
int Book::getId() const{
return this->id;
}
```
##### 用户类 `User.h` 和 `User.cpp`
这里仅展示头文件部分:
```cpp
#ifndef USER_H_
#define USER_H_
enum class Role { ADMINISTRATOR, LIBRARIAN, MEMBER };
class User {
private:
std::string name;
Role role;
protected:
bool canBorrowBooks();
public:
explicit User(std::string n, Role r);
virtual ~User();
const std::string& getName() const;
Role getRole() const;
};
#endif /*USER_H_*/
```
以上展示了如何利用面向对象特性来创建基本实体模型——即书籍(`Book`)和用户(`User`)两个主要组件之间的关系建模[^2]。
对于完整的项目来说,还需要加入更多细节如数据库连接逻辑、异常处理策略等方面的内容,在此不再赘述。
阅读全文
相关推荐



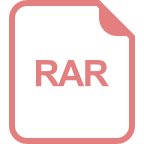


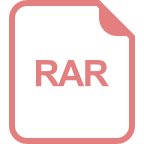
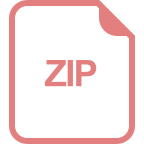










