java后端中如何发送post请求
时间: 2024-12-22 14:24:55 浏览: 15
在Java后端发送POST请求通常使用HTTP客户端库,如`HttpURLConnection`, `Apache HttpClient`, 或者第三方库如`Spring RestTemplate`, `OkHttp`, 和 `Retrofit`。这里我会介绍使用`HttpURLConnection`和`Spring RestTemplate`的例子。
**使用HttpURLConnection发送POST请求(同步):**
```java
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class PostRequestExample {
public static void main(String[] args) throws Exception {
String url = "http://example.com/api/endpoint";
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
// 设置为POST请求
con.setRequestMethod("POST");
// 添加请求参数,假设参数名是"key", 值是"value"
String params = "key=value";
byte[] paramBytes = params.getBytes();
con.setDoOutput(true); // 需要设置输出流
OutputStream os = con.getOutputStream();
os.write(paramBytes);
os.flush();
os.close();
int responseCode = con.getResponseCode();
System.out.println("Response Code : " + responseCode);
// 读取响应
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
}
}
```
**使用Spring RestTemplate发送POST请求(异步或同步):**
```java
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
public class SpringPostRequestExample {
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate(); // 创建RestTemplate实例
// 使用POST请求
String apiUrl = "http://example.com/api/endpoint";
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_FORM_URLENCODED);
HttpEntity<String> request = new HttpEntity<>("key=value", headers);
ResponseEntity<String> response = restTemplate.postForEntity(apiUrl, request, String.class);
System.out.println("Response Status: " + response.getStatusCode());
System.out.println("Response Body: " + response.getBody());
}
}
```
以上代码片段展示了基本的POST请求操作。根据项目需求,你可以选择适合的方式并进行相应的错误处理、异常处理以及添加认证信息等。
阅读全文
相关推荐
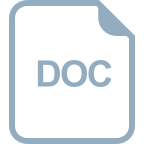
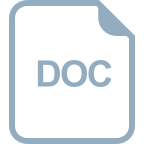
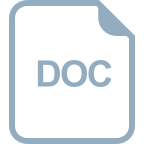
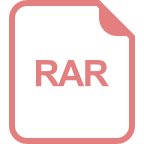
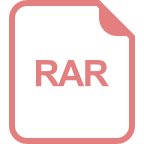
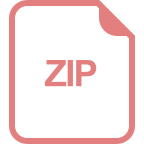
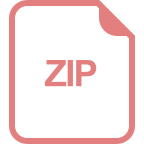
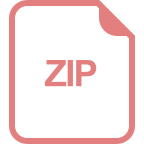
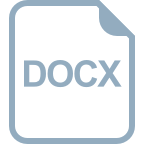
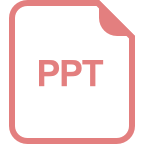
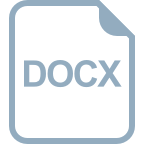
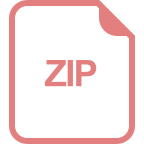
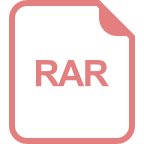
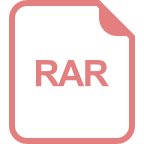
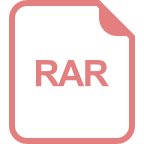
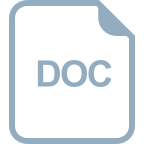


