import pandas as pd df = pd.read_csv('file.csv') df.loc[2, 'column_name'] = 100是什么意思
时间: 2024-05-19 18:12:10 浏览: 12
这段代码使用 pandas 库读取名为 'file.csv' 的 CSV 文件,并将第 2 行中 'column_name' 列的值更改为 100。其中,'column_name' 是 CSV 文件中的一列名称。这意味着该文件中的第 3 行(由于 Python 中的行索引从 0 开始)的 'column_name' 列的值将被更改为 100。
相关问题
python读取csv文件并修改指定内容-pandas读取CSV文件时查看修改各列的数据类型格式...
可以使用 Pandas 库来读取 CSV 文件并修改指定内容。以下是一个示例代码:
```python
import pandas as pd
# 读取 CSV 文件
df = pd.read_csv('file.csv')
# 查看数据类型
print(df.dtypes)
# 修改指定内容
df.loc[df['Column_Name'] == 'Value_to_Be_Changed', 'Column_Name'] = 'New_Value'
# 修改数据类型
df['Column_Name'] = df['Column_Name'].astype('New_Data_Type')
# 保存修改后的 CSV 文件
df.to_csv('file_modified.csv', index=False)
```
其中,`Column_Name` 是需要修改的列名,`Value_to_Be_Changed` 是需要修改的指定内容,`New_Value` 是修改后的值,`New_Data_Type` 是修改后的数据类型。修改后的 CSV 文件将保存在 `file_modified.csv` 中。
pandas读取csv文件
### 回答1:
可以使用 Pandas 库中的 `read_csv()` 函数读取 CSV 文件。以下是读取 CSV 文件并将其存储在 Pandas 数据帧(DataFrame)中的示例代码:
```python
import pandas as pd
df = pd.read_csv('filename.csv')
```
其中,`filename.csv` 是你要读取的 CSV 文件名。你可以根据需要传递其他参数,例如分隔符、列名等。
### 回答2:
Pandas是一个强大的数据分析工具,可以通过其提供的read_csv()函数来读取CSV文件。
读取CSV文件的基本步骤如下:
首先,需要导入pandas库,一般习惯使用`import pandas as pd`来导入,并将其简称为pd。
然后,使用`pd.read_csv()`函数来读取CSV文件。该函数的参数包括文件的路径和文件名,例如:
``` python
df = pd.read_csv("file.csv")
```
其中,file.csv是要读取的CSV文件的路径和文件名。可以使用绝对路径或者相对路径。
接下来,可以对读取到的数据进行一些基本操作,例如查看数据的前几行、数据的维度、数据的列名等。
通过`df.head()`函数可以查看数据的前几行,默认情况下是前5行。例如:
``` python
print(df.head())
```
通过`df.shape`可以查看数据的维度,即行数和列数。例如:
``` python
print(df.shape)
```
通过`df.columns`可以查看数据的列名。例如:
``` python
print(df.columns)
```
最后,可以通过使用pandas提供的数据分析和处理功能对读取到的数据进行进一步处理和分析。
以上是使用pandas读取CSV文件的基本步骤和一些常用操作。读取过程中,可能会遇到一些特殊情况,需要根据具体情况进行调整。
### 回答3:
Pandas是一个强大的数据处理和分析库,可以轻松读取各种格式的数据,包括CSV文件。下面是一个使用Pandas读取CSV文件的示例:
```python
import pandas as pd
# 读取CSV文件
data = pd.read_csv('data.csv')
# 显示数据的前几行
print(data.head())
# 获取数据的行数和列数
num_rows, num_cols = data.shape
print(f"数据有 {num_rows} 行和 {num_cols} 列")
# 获取数据的列名
column_names = data.columns
print("数据的列名: ", column_names)
# 获取指定列的数据
column_data = data['column_name']
print("列的数据: ", column_data)
# 获取指定行的数据
row_data = data.loc[0]
print("行的数据: ", row_data)
```
在上面的代码中,我们首先导入了`pandas`库,并使用`read_csv`函数将CSV文件读取为一个Pandas的数据帧(DataFrame)。然后,我们可以使用`head`方法来显示数据的前几行,使用`shape`方法来获取数据的行数和列数,使用`columns`属性来获取列名,使用`loc`方法来获取指定行的数据,使用索引或列名来获取指定列的数据。
希望这个简短的回答能够帮助您了解如何使用Pandas读取CSV文件。
相关推荐
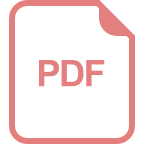













