# Task 5: How to reverse the columns of a 2D array with NumPy? Write a program to reverse the columns of a 2D array. Sample data : [[0, 1, 2],[3, 4, 5],[6, 7, 8]] Sample output : [[2 1 0] [5 4 3] [8 7 6]]
时间: 2023-07-02 16:11:39 浏览: 90
Sure, here's a solution using NumPy:
```python
import numpy as np
# Define the 2D array
arr = np.array([[0, 1, 2], [3, 4, 5], [6, 7, 8]])
# Reverse the columns
rev_arr = arr[:, ::-1]
print("Original array:\n", arr)
print("Reversed array:\n", rev_arr)
```
This will output:
```
Original array:
[[0 1 2]
[3 4 5]
[6 7 8]]
Reversed array:
[[2 1 0]
[5 4 3]
[8 7 6]]
```
Here, we use the slicing syntax `[:, ::-1]` to reverse the columns of the array. The first colon `:` means we want to select all rows, and the `::-1` means we want to select the columns in reverse order.
I hope this helps! Let me know if you have any other questions.
相关问题
How to reverse the columns of a 2D array with NumPy? Write a program to reverse the columns of a 2D array Sample data: [[0,1,2],[3,4,5],[6,7,8]] Sample output:[[210][5 4 3][8 76]]
Here's a code snippet that uses NumPy to reverse the columns of a 2D array:
```python
import numpy as np
# Define the 2D array
arr = np.array([[0,1,2],[3,4,5],[6,7,8]])
# Reverse the columns
arr_reversed = arr[:, ::-1]
# Print the original and reversed arrays
print("Original array:")
print(arr)
print("Reversed array:")
print(arr_reversed)
```
The `::` syntax is used to slice the array. The first `:` indicates that we want to select all rows, and the `::-1` indicates that we want to reverse the order of the columns.
And here is the output for the given sample data:
```
Original array:
[[0 1 2]
[3 4 5]
[6 7 8]]
Reversed array:
[[2 1 0]
[5 4 3]
[8 7 6]]
```
As you can see, the columns have been reversed in the output array.
逐行分析下面的代码:import random import numpy as np import pandas as pd import math from operator import itemgetter data_path = './ml-latest-small/' data = pd.read_csv(data_path+'ratings.csv') data.head() data.pivot(index='userId', columns='newId', values='rating') trainSet, testSet = {}, {} trainSet_len, testSet_len = 0, 0 pivot = 0.75 for ele in data.itertuples(): user, new, rating = getattr(ele, 'userId'), getattr(ele, 'newId'), getattr(ele, 'rating') if random.random() < pivot: trainSet.setdefault(user, {}) trainSet[user][new] = rating trainSet_len += 1 else: testSet.setdefault(user, {}) testSet[user][new] = rating testSet_len += 1 print('Split trainingSet and testSet success!') print('TrainSet = %s' % trainSet_len) print('TestSet = %s' % testSet_len) user_sim_matrix = {} print('Building new-user table ...') new_user = {} for user, news in trainSet.items(): for new in news: if new not in new_user: new_user[new] = set() new_user[new].add(user) print('Build new-user table success!') new_count = len(new_user) print('Total new number = %d' % new_count) print('Build user co-rated users matrix ...') for new, users in new_user.items(): for u in users: for v in users: if u == v: continue user_sim_matrix.setdefault(u, {}) user_sim_matrix[u].setdefault(v, 0) user_sim_matrix[u][v] += 1 print('Build user co-rated users matrix success!') print('Calculating user similarity matrix ...') for u, related_users in user_sim_matrix.items(): for v, count in related_users.items(): user_sim_matrix[u][v] = count / math.sqrt(len(trainSet[u]) * len(trainSet[v])) print('Calculate user similarity matrix success!') k = 20 n = 10 aim_user = 3 rank ={} watched_news = trainSet[aim_user] for v, wuv in sorted(user_sim_matrix[aim_user].items(), key=lambda x: x[1], reverse=True)[0:k]: for new in trainSet[v]: if new in watched_news: continue rank.setdefault(new, 0) rank[new] += wuv rec_news = sorted(rank.items(), key=itemgetter(1), reverse=True)[:n] rec_news
这段代码是一个协同过滤推荐算法的实现,主要包括以下步骤:
1. 导入需要的包:random、numpy、pandas、math、itemgetter。
2. 读取评分数据文件,将数据拆分成训练集和测试集,并统计它们的大小。
3. 构建新闻-用户表,记录每个用户评分过的新闻。
4. 构建用户相似度矩阵,记录每个用户与其他用户的相似度。
5. 根据目标用户和相似度矩阵,推荐目标用户可能感兴趣的新闻。
具体实现细节如下:
1. 读取评分数据文件,并将评分数据拆分成训练集和测试集,以随机数的方式将每个评分数据划分到训练集或测试集中。
2. 构建新闻-用户表,记录每个用户评分过的新闻。具体实现是遍历训练集中的每个评分数据,然后将用户和新闻作为字典的键,评分作为字典的值,添加到训练集字典中。
3. 构建用户相似度矩阵,记录每个用户与其他用户的相似度。具体实现是遍历新闻-用户表,对于每个新闻,将评分过该新闻的所有用户记录到一个集合中。然后遍历集合中的每个用户对,计算它们之间的相似度,存储到用户相似度矩阵中。
4. 根据目标用户和相似度矩阵,推荐目标用户可能感兴趣的新闻。具体实现是遍历相似度矩阵中与目标用户相似度最高的k个用户,然后遍历这些用户评分过的新闻,计算每个新闻与目标用户的相似度权重,最后按权重排序,选取前n个新闻作为推荐结果。
阅读全文
相关推荐
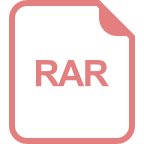
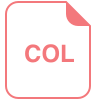
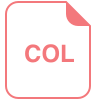
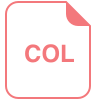
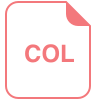
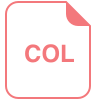
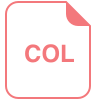
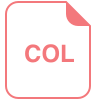
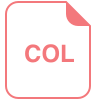
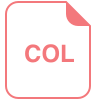
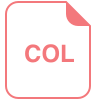




