opencv dlib python大眼代码
时间: 2023-07-10 14:29:54 浏览: 251
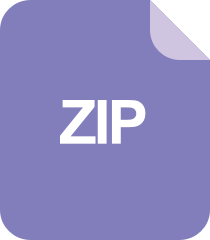
基于Python+OpenCVdlib的疲劳驾驶检测系统源码+数据集+详细文档(高分毕业设计).zip

以下是使用 OpenCV 和 dlib 库在 Python 中实现大眼效果的示例代码:
```python
import cv2
import dlib
# 加载人脸关键点检测模型
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 加载图像
img = cv2.imread("example.jpg")
# 检测人脸
faces = detector(img, 1)
# 遍历每个人脸
for face in faces:
# 提取人脸关键点
landmarks = predictor(img, face)
left_eye = []
right_eye = []
for i in range(36, 42):
left_eye.append((landmarks.part(i).x, landmarks.part(i).y))
for i in range(42, 48):
right_eye.append((landmarks.part(i).x, landmarks.part(i).y))
# 计算眼睛中心点
left_eye_center = (int((left_eye[0][0] + left_eye[3][0]) / 2), int((left_eye[1][1] + left_eye[4][1]) / 2))
right_eye_center = (int((right_eye[0][0] + right_eye[3][0]) / 2), int((right_eye[1][1] + right_eye[4][1]) / 2))
# 计算眼睛半径
left_eye_radius = int(((left_eye[3][0] - left_eye[0][0]) + (left_eye[4][1] - left_eye[1][1])) / 4)
right_eye_radius = int(((right_eye[3][0] - right_eye[0][0]) + (right_eye[4][1] - right_eye[1][1])) / 4)
# 模拟大眼效果
# 扩大眼睛的半径
left_eye_radius += int(left_eye_radius * 0.2)
right_eye_radius += int(right_eye_radius * 0.2)
# 绘制眼睛
cv2.circle(img, left_eye_center, left_eye_radius, (0, 255, 0), -1)
cv2.circle(img, right_eye_center, right_eye_radius, (0, 255, 0), -1)
# 显示图像
cv2.imshow("image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
需要注意的是,此代码需要依赖以下库:
- OpenCV
- dlib
- numpy
而且需要提前下载并将 `shape_predictor_68_face_landmarks.dat` 文件放在代码同级目录下,该文件是 dlib 提供的人脸关键点检测模型。
阅读全文
相关推荐
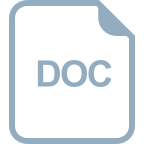
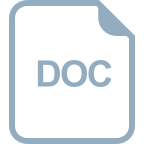
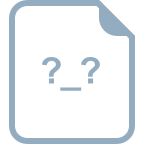
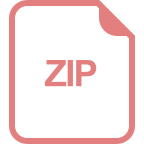
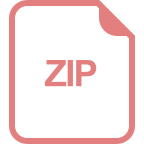
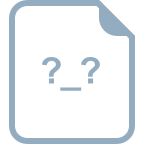
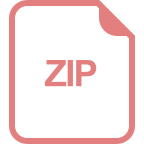
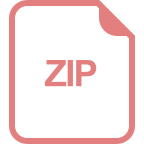
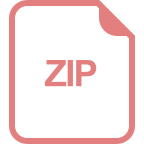
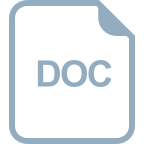
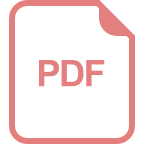
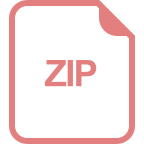
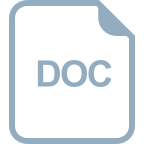
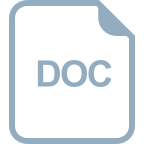


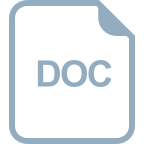
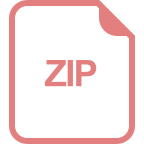
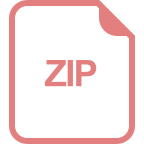