用dlib和opencv函数以python语言实现放大人眼的功能
时间: 2024-03-03 07:52:09 浏览: 113
以下是使用Dlib和OpenCV函数以Python实现放大人眼的完整代码:
```python
import cv2
import dlib
import numpy as np
# 加载预训练的人脸关键点检测器
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 加载人脸检测器
detector = dlib.get_frontal_face_detector()
# 加载图像
img = cv2.imread("input.jpg")
# 将图像转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = detector(gray)
# 遍历每个检测到的人脸
for face in faces:
# 获取人脸的关键点
landmarks = predictor(gray, face)
# 获取左眼和右眼的关键点坐标
left_eye = np.array([(landmarks.part(36).x, landmarks.part(36).y),
(landmarks.part(37).x, landmarks.part(37).y),
(landmarks.part(38).x, landmarks.part(38).y),
(landmarks.part(39).x, landmarks.part(39).y),
(landmarks.part(40).x, landmarks.part(40).y),
(landmarks.part(41).x, landmarks.part(41).y)], np.int32)
right_eye = np.array([(landmarks.part(42).x, landmarks.part(42).y),
(landmarks.part(43).x, landmarks.part(43).y),
(landmarks.part(44).x, landmarks.part(44).y),
(landmarks.part(45).x, landmarks.part(45).y),
(landmarks.part(46).x, landmarks.part(46).y),
(landmarks.part(47).x, landmarks.part(47).y)], np.int32)
# 计算左眼和右眼的中心坐标
left_eye_center = np.mean(left_eye, axis=0).astype(np.int32)
right_eye_center = np.mean(right_eye, axis=0).astype(np.int32)
# 计算左眼和右眼的距离
eye_distance = np.linalg.norm(left_eye_center - right_eye_center)
# 计算调整后的左眼和右眼的坐标
left_eye_adjusted = left_eye + (right_eye_center - left_eye_center) * 0.15 * eye_distance / np.linalg.norm(left_eye - right_eye)
right_eye_adjusted = right_eye + (left_eye_center - right_eye_center) * 0.15 * eye_distance / np.linalg.norm(right_eye - left_eye)
# 创建一个黑色的掩膜
mask = np.zeros((img.shape[0], img.shape[1]), dtype=np.uint8)
# 填充左眼和右眼的区域
cv2.fillPoly(mask, [left_eye_adjusted], 255)
cv2.fillPoly(mask, [right_eye_adjusted], 255)
# 对图像进行腐蚀操作,使填充区域缩小
kernel = np.ones((5, 5), np.uint8)
mask = cv2.erode(mask, kernel, iterations=1)
# 对图像进行高斯模糊
mask = cv2.GaussianBlur(mask, (11, 11), 0)
# 计算调整后的左眼和右眼的半径
left_eye_radius = int(0.4 * eye_distance)
right_eye_radius = int(0.4 * eye_distance)
# 对图像进行放大处理
left_eye_zoomed = cv2.resize(img[left_eye_center[1]-left_eye_radius:left_eye_center[1]+left_eye_radius, left_eye_center[0]-left_eye_radius:left_eye_center[0]+left_eye_radius], None, fx=2.5, fy=2.5)
right_eye_zoomed = cv2.resize(img[right_eye_center[1]-right_eye_radius:right_eye_center[1]+right_eye_radius, right_eye_center[0]-right_eye_radius:right_eye_center[0]+right_eye_radius], None, fx=2.5, fy=2.5)
# 将放大后的图像粘贴回原图上
img[left_eye_center[1]-left_eye_radius:left_eye_center[1]+left_eye_radius, left_eye_center[0]-left_eye_radius:left_eye_center[0]+left_eye_radius] = left_eye_zoomed
img[right_eye_center[1]-right_eye_radius:right_eye_center[1]+right_eye_radius, right_eye_center[0]-right_eye_radius:right_eye_center[0]+right_eye_radius] = right_eye_zoomed
# 显示处理后的图像
cv2.imshow("output", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请确保`shape_predictor_68_face_landmarks.dat`文件与Python脚本位于同一目录中,并且将代码中的`input.jpg`替换为您要处理的图像的文件名。运行代码后,将会显示处理后的图像,眼睛区域将会被放大。
阅读全文
相关推荐
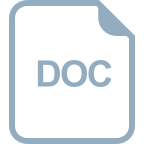
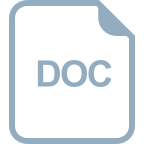
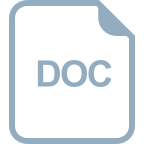
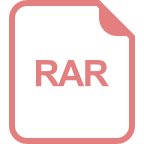
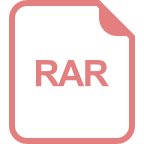
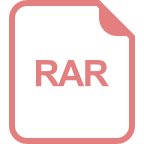
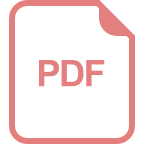
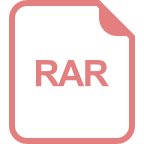
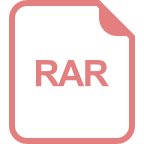
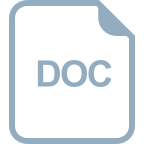
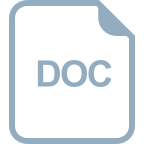

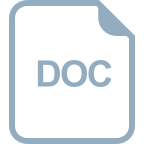
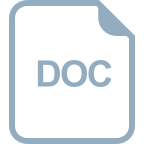
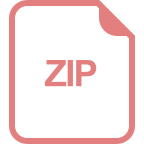
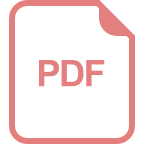
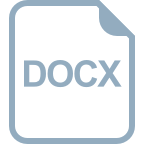