写一个ESP32C3芯片通过wifi连接采集网络时间并显示在0.96寸i2c通讯的oled显示屏上
时间: 2024-05-16 14:18:02 浏览: 16
首先,需要使用ESP-IDF开发环境,包括ESP32C3的SDK和相关工具链。
接下来,按照以下步骤进行操作:
1. 配置WiFi连接:在ESP-IDF中,使用WiFi连接需要配置一个WiFi连接管理器。在代码中添加以下代码:
```c
#include "esp_wifi.h"
#include "esp_event.h"
#include "esp_log.h"
#define WIFI_SSID "your_wifi_ssid"
#define WIFI_PASS "your_wifi_password"
static void wifi_event_handler(void* arg, esp_event_base_t event_base, int32_t event_id, void* event_data) {
if (event_id == WIFI_EVENT_STA_START) {
esp_wifi_connect();
} else if (event_id == WIFI_EVENT_STA_DISCONNECTED) {
esp_wifi_connect();
}
}
void wifi_init_sta(void) {
tcpip_adapter_init();
ESP_ERROR_CHECK(esp_event_loop_create_default());
wifi_init_config_t cfg = WIFI_INIT_CONFIG_DEFAULT();
ESP_ERROR_CHECK(esp_wifi_init(&cfg));
ESP_ERROR_CHECK(esp_event_handler_register(WIFI_EVENT, ESP_EVENT_ANY_ID, &wifi_event_handler, NULL));
wifi_config_t wifi_config = {
.sta = {
.ssid = WIFI_SSID,
.password = WIFI_PASS,
},
};
ESP_ERROR_CHECK(esp_wifi_set_mode(WIFI_MODE_STA));
ESP_ERROR_CHECK(esp_wifi_set_config(ESP_IF_WIFI_STA, &wifi_config));
ESP_ERROR_CHECK(esp_wifi_start());
}
```
2. 获取网络时间:在ESP32C3上获取网络时间需要使用NTP协议。在代码中添加以下代码:
```c
#include "esp_sntp.h"
void obtain_time(void) {
ESP_LOGI(TAG, "Obtaining time from NTP server...");
sntp_setoperatingmode(SNTP_OPMODE_POLL);
sntp_setservername(0, "pool.ntp.org");
sntp_init();
// wait for time to be set
time_t now = 0;
struct tm timeinfo = {0};
while (timeinfo.tm_year < (2021 - 1900)) {
ESP_LOGI(TAG, "Waiting for system time to be set...");
vTaskDelay(1000 / portTICK_PERIOD_MS);
time(&now);
localtime_r(&now, &timeinfo);
}
}
```
3. 显示时间:接下来,将获取的时间显示在OLED屏幕上。在代码中添加以下代码:
```c
#include "driver/i2c.h"
#include "ssd1306.h"
#define I2C_SDA_GPIO 21
#define I2C_SCL_GPIO 22
ssd1306_t dev;
void oled_init(void) {
i2c_config_t i2c_config = {
.mode = I2C_MODE_MASTER,
.sda_io_num = I2C_SDA_GPIO,
.scl_io_num = I2C_SCL_GPIO,
.sda_pullup_en = GPIO_PULLUP_ENABLE,
.scl_pullup_en = GPIO_PULLUP_ENABLE,
.master.clk_speed = 1000000,
};
ESP_ERROR_CHECK(i2c_param_config(I2C_NUM_0, &i2c_config));
ESP_ERROR_CHECK(i2c_driver_install(I2C_NUM_0, I2C_MODE_MASTER, 0, 0, 0));
ssd1306_init(&dev, 128, 64, ssd1306_i2c_addr);
ssd1306_set_whole_display_lighting(&dev, false);
ssd1306_clear_screen(&dev, false);
}
void show_time(struct tm* timeinfo) {
char strftime_buf[64];
strftime(strftime_buf, sizeof(strftime_buf), "%c", timeinfo);
ssd1306_clear_screen(&dev, false);
ssd1306_draw_string(&dev, 0, 0, strftime_buf, 1, true);
ssd1306_refresh(&dev, true);
}
```
4. 将上述步骤集成到主函数中:
```c
void app_main() {
wifi_init_sta();
obtain_time();
oled_init();
struct tm timeinfo = {0};
time_t now = 0;
while (1) {
time(&now);
localtime_r(&now, &timeinfo);
show_time(&timeinfo);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
```
这样,ESP32C3就可以连接WiFi,获取网络时间,并将时间显示在OLED屏幕上了。
相关推荐
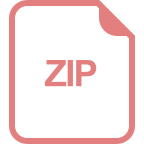
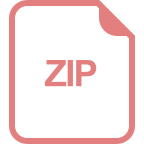














