用Python实现数据库可视化
时间: 2023-11-29 19:14:26 浏览: 75
可以使用Python中的Tkinter模块和SQLite数据库来实现简单的数据库可视化。以下是一个简单的示例代码:
```python
import tkinter as tk
import sqlite3
# 创建GUI窗口
root = tk.Tk()
root.title("数据库可视化")
# 连接SQLite数据库
conn = sqlite3.connect("mydatabase.db")
c = conn.cursor()
# 创建表格
c.execute('''CREATE TABLE IF NOT EXISTS mytable
(id INTEGER PRIMARY KEY,
name TEXT,
age INTEGER)''')
# 插入数据
c.execute("INSERT INTO mytable (name, age) VALUES (?, ?)", ("Alice", 25))
c.execute("INSERT INTO mytable (name, age) VALUES (?, ?)", ("Bob", 30))
c.execute("INSERT INTO mytable (name, age) VALUES (?, ?)", ("Charlie", 35))
conn.commit()
# 查询数据
c.execute("SELECT * FROM mytable")
results = c.fetchall()
# 显示数据
for i, row in enumerate(results):
tk.Label(root, text=row[0]).grid(row=i+1, column=0)
tk.Label(root, text=row[1]).grid(row=i+1, column=1)
tk.Label(root, text=row[2]).grid(row=i+1, column=2)
# 关闭数据库连接
conn.close()
# 运行GUI程序
root.mainloop()
```
这个示例代码会在GUI窗口中创建一个简单的表格,并在其中显示一个SQLite数据库中的数据。你可以根据自己的需要修改这个示例代码,来实现自己的数据库可视化应用。
阅读全文
相关推荐
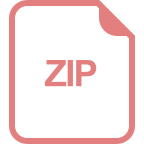
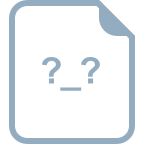


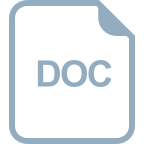
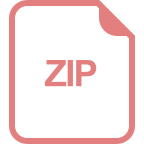
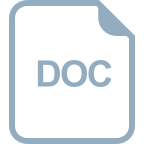
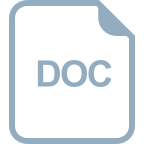


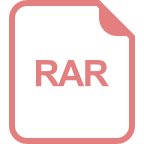
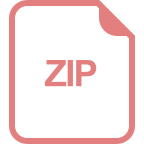
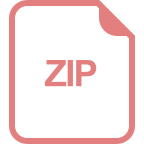
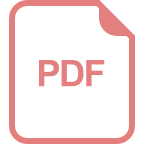
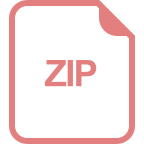
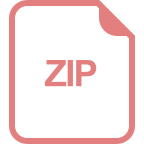
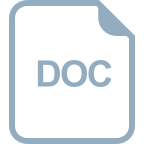
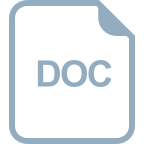