const columns: ProColumns<API.BatteryInformationControl>[] = [ { title: ( "截止日期" ), dataIndex: 'dataEndDate', fixed:'left', align: "center", width: 150, }, ]; return ( <div> <Space direction="vertical" size="large" style={{display: 'flex', marginTop: '1%'}}> <div> <span> 日期: </span> <DatePicker style={{width: '20%'}} onChange={onChange} disabledDate={disabledDate} defaultValue={dayjs()} /> </div> <ProTable<API.BatteryInformationControl, API.queryBatteryInformationControlParams & API.PageParams > headerTitle={'深圳市能源换电业务电池总体情况'} rowKey="" bordered // search={false} // scroll={{x:true}} scroll={{x: 'max-content', y: 700}} request={async (params) => { console.log(params); const body = { ...params, dataQueryTime: selectedDate } delete body.current; delete body.pageSize; console.log(body); const res = await queryBatteryInformationManagement(body); console.log(res); setSummaryData(res) return res }} options={{ // show: true, density: true, fullScreen: true, setting: true, } } columns={columns} summary={ () => ( <> <ProTable.Summary.Row className='total' style={{textAlign: 'center'}}> {/* 合计行内容 */} <ProTable.Summary.Cell colSpan={2} index={0} >总计</ProTable.Summary.Cell> <ProTable.Summary.Cell index={2}>{summaryData && summaryData.total}</ProTable.Summary.Cell> </ProTable.Summary.Row> </> )} /> </Space> </div> )怎么把protable的截止时间搜索框的值改为一个时间选择器
时间: 2024-04-14 15:25:46 浏览: 18
要将ProTable的截止时间搜索框的值改为一个时间选择器,您可以按照以下步骤进行操作:
1. 修改`columns`数组,将截止日期的列定义中的`dataIndex`字段更改为一个唯一的键,例如`'dataEndDate'`改为`'endDate'`。
```jsx
const columns: ProColumns<API.BatteryInformationControl>[] = [
{
title: "截止日期",
dataIndex: 'endDate', // 修改dataIndex字段为endDate
fixed: 'left',
align: "center",
width: 150,
},
// 其他列定义
];
```
2. 在组件中创建一个新的状态变量来存储截止时间的值。例如,您可以使用useState钩子创建一个名为`selectedEndDate`的状态变量,并在时间选择器的onChange事件处理程序中更新它。
```jsx
const [selectedEndDate, setSelectedEndDate] = useState(dayjs());
const onEndDateChange = (date) => {
setSelectedEndDate(date);
};
```
3. 将时间选择器添加到组件的JSX中,并将其与新的状态变量和onChange事件处理程序关联。
```jsx
<DatePicker
style={{ width: '20%' }}
onChange={onEndDateChange}
defaultValue={selectedEndDate}
/>
```
4. 在ProTable的request属性中,将`selectedEndDate`的值添加到请求参数对象中的相应字段中。根据您提供的代码,可以将其添加到`dataQueryTime`字段中。
```jsx
request={async (params) => {
const body = {
...params,
dataQueryTime: selectedEndDate.format('YYYY-MM-DD'), // 将selectedEndDate的值添加到请求参数中
};
delete body.current;
delete body.pageSize;
// 发起请求并返回结果
const res = await queryBatteryInformationManagement(body);
setSummaryData(res);
return res;
}}
```
最终的代码示例:
```jsx
import { useState } from 'react';
import { DatePicker, ProTable } from 'antd';
const YourComponent = () => {
const [selectedEndDate, setSelectedEndDate] = useState(dayjs());
const onEndDateChange = (date) => {
setSelectedEndDate(date);
};
const columns: ProColumns<API.BatteryInformationControl>[] = [
{
title: "截止日期",
dataIndex: 'endDate',
fixed: 'left',
align: "center",
width: 150,
},
// 其他列定义
];
return (
<div>
<DatePicker
style={{ width: '20%' }}
onChange={onEndDateChange}
defaultValue={selectedEndDate}
/>
<ProTable
// ...其他属性
request={async (params) => {
const body = {
...params,
dataQueryTime: selectedEndDate.format('YYYY-MM-DD'),
};
delete body.current;
delete body.pageSize;
// 发起请求并返回结果
const res = await queryBatteryInformationManagement(body);
setSummaryData(res);
return res;
}}
>
{columns.map((column) => (
<ProTable.Column {...column} />
))}
</ProTable>
</div>
);
};
export default YourComponent;
```
请根据您的代码结构和需求进行相应的修改。希望对您有所帮助!
相关推荐
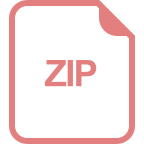
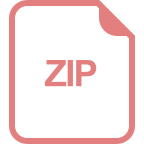
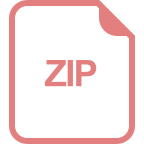















