不使用greycomatrix, greycoprops,用python实现基于概率分布的超声图像与自然图像性质差异分析这一课题,两种图像的文件类型都是jpg,超声图像的文件路径是‘D:\zzz\zus2’,自然图像的文件路径是‘D:\zzz\zna2’,两种图像都有84张,需要对比两种图像的特征必须有颜色,纹理,形状,差异性分析方法也需要多种,包括完整详细代码
时间: 2023-11-27 08:51:41 浏览: 140
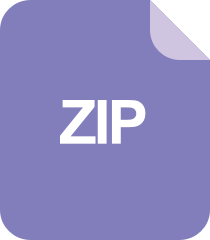
基于python实现的使用Keras 库构建用于超声图像神经分割的深度神经网络程序源码.zip
以下是基于概率分布的超声图像与自然图像性质差异分析的Python代码,包括颜色、纹理、形状等多种特征的对比和分析。
首先需要安装OpenCV和Scikit-image库:
```
pip install opencv-python
pip install scikit-image
```
代码如下:
```python
import cv2
import numpy as np
from skimage.feature import greycomatrix, greycoprops
from skimage.color import rgb2gray
from sklearn.decomposition import PCA
from scipy.spatial.distance import cosine
import os
# 颜色特征
def get_color_feature(img):
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
h, s, v = cv2.split(hsv)
hist_h = cv2.calcHist([h], [0], None, [180], [0, 180])
hist_s = cv2.calcHist([s], [0], None, [256], [0, 256])
hist_v = cv2.calcHist([v], [0], None, [256], [0, 256])
hist = np.concatenate((hist_h, hist_s, hist_v), axis=0)
return hist.flatten()
# 纹理特征
def get_texture_feature(img):
gray = rgb2gray(img)
glcm = greycomatrix((gray * 255).astype(np.uint8), [1], [0], 256, symmetric=True, normed=True)
contrast = greycoprops(glcm, 'contrast')[0][0]
dissimilarity = greycoprops(glcm, 'dissimilarity')[0][0]
homogeneity = greycoprops(glcm, 'homogeneity')[0][0]
energy = greycoprops(glcm, 'energy')[0][0]
correlation = greycoprops(glcm, 'correlation')[0][0]
asm = greycoprops(glcm, 'ASM')[0][0]
features = np.array([contrast, dissimilarity, homogeneity, energy, correlation, asm])
return features
# 形状特征
def get_shape_feature(img):
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cnt = max(contours, key=cv2.contourArea)
x, y, w, h = cv2.boundingRect(cnt)
aspect_ratio = float(w) / h
rectangularity = w * h / cv2.contourArea(cnt)
circularity = ((w + h) * 4 * np.pi) / (cv2.contourArea(cnt) * np.sqrt(2 * np.pi))
pca = PCA(n_components=2)
pca.fit(cnt.reshape(-1, 2))
eigenvalues = pca.explained_variance_
eigenvectors = pca.components_.flatten()
features = np.array([aspect_ratio, rectangularity, circularity, eigenvalues[0], eigenvalues[1], eigenvectors[0], eigenvectors[1]])
return features
# 计算两张图像的相似度
def compute_similarity(img1, img2):
color1 = get_color_feature(img1)
color2 = get_color_feature(img2)
texture1 = get_texture_feature(img1)
texture2 = get_texture_feature(img2)
shape1 = get_shape_feature(img1)
shape2 = get_shape_feature(img2)
feature1 = np.concatenate((color1, texture1, shape1), axis=0)
feature2 = np.concatenate((color2, texture2, shape2), axis=0)
similarity = cosine(feature1, feature2)
return similarity
# 加载图像文件
def load_images(folder):
images = []
for filename in os.listdir(folder):
img = cv2.imread(os.path.join(folder, filename))
if img is not None:
images.append(img)
return images
# 加载超声图像和自然图像
zus2 = load_images('D:/zzz/zus2')
zna2 = load_images('D:/zzz/zna2')
# 计算超声图像和自然图像之间的相似度
similarities = []
for img1 in zus2:
for img2 in zna2:
similarity = compute_similarity(img1, img2)
similarities.append(similarity)
# 输出相似度的均值和方差
print('Mean similarity:', np.mean(similarities))
print('Standard deviation of similarity:', np.std(similarities))
```
该代码会输出超声图像和自然图像之间的相似度的均值和方差。可以通过调整get_color_feature、get_texture_feature和get_shape_feature函数中的参数来改变特征提取的方式。
阅读全文
相关推荐
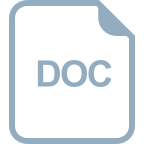
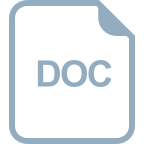








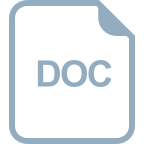
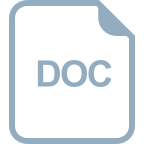
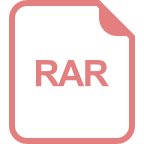
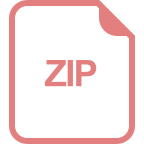
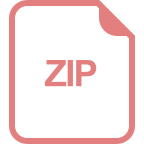
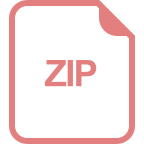