python如何画出速度时间曲线
时间: 2023-07-20 20:24:23 浏览: 125
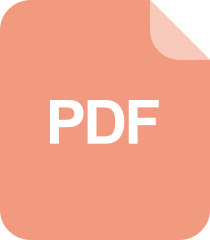
使用python动态生成波形曲线的实现
在Python中,可以使用Matplotlib库来画出速度时间曲线。以下是一个简单的示例代码,供你参考:
```python
import matplotlib.pyplot as plt
# 定义时间和速度数据
time = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
speed = [0, 10, 20, 30, 40, 50, 60, 70, 80, 90]
# 绘制速度时间曲线
plt.plot(time, speed)
# 添加标题和坐标轴标签
plt.title('Speed-Time Curve')
plt.xlabel('Time (s)')
plt.ylabel('Speed (km/h)')
# 显示图形
plt.show()
```
运行上述代码后,即可显示出速度时间曲线的图形。你可以根据自己的需求,修改时间和速度的数据,以及图形的标题和标签。
阅读全文
相关推荐
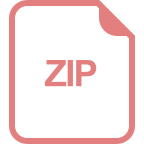
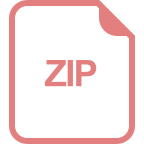















