python application/x-www-form-urlencoded; charset=utf-8格式的参数如何传入post请求
时间: 2023-05-31 19:19:46 浏览: 100
### 回答1:
Python中可以使用requests库发送POST请求,并且可以通过data参数传递application/x-www-form-urlencoded格式的参数。具体方法如下:
1. 导入requests库
```python
import requests
```
2. 构造请求参数
```python
data = {
'key1': 'value1',
'key2': 'value2'
}
```
3. 发送POST请求
```python
url = 'http://example.com/api'
response = requests.post(url, data=data)
```
其中,url为请求的URL地址,data为请求参数。requests.post()方法会自动将data参数转换为application/x-www-form-urlencoded格式的参数,并添加到请求体中发送。如果需要设置请求头,可以使用headers参数,例如:
```python
headers = {
'Content-Type': 'application/x-www-form-urlencoded; charset=utf-8'
}
response = requests.post(url, data=data, headers=headers)
```
这样就可以发送application/x-www-form-urlencoded格式的POST请求了。
### 回答2:
在Python中,使用requests库发送POST请求时,可以通过data参数来传入application/x-www-form-urlencoded; charset=utf-8格式的参数。具体的方法如下:
1. 导入requests库
在Python代码中导入requests库,即可使用其中的相关方法发送HTTP请求。
```
import requests
```
2. 构造POST请求参数
构造POST请求参数时,需要将参数封装成一个字典。
```
data = {
'param1': 'value1',
'param2': 'value2',
'param3': 'value3'
}
```
在这里,我们设定了三个参数,分别为param1、param2和param3,分别对应的值为value1、value2和value3。
3. 发送POST请求
使用requests库的post方法发送POST请求。
```
response = requests.post(url, data=data)
```
其中,url指的是发送POST请求的目标地址,data是需要发送的参数,如果需要发送headers和cookies等信息,也可以一并传入到post方法中。
4. 处理响应结果
发送POST请求后,服务器会返回一个响应对象,我们可以通过访问这个对象的属性来获取响应结果。
```
print(response.status_code) # 获取响应状态码
print(response.text) # 获取响应正文
```
由于我们发送的是application/x-www-form-urlencoded; charset=utf-8格式的参数,服务器返回的响应正文也是同样的格式。如果需要将其转换成Python字典,可以使用urllib库的parse_qs方法进行解析。
```
from urllib.parse import parse_qs
response_dict = parse_qs(response.text)
print(response_dict)
```
在这里,我们将服务器返回的响应正文解析成了Python字典,方便后续的数据处理。
### 回答3:
在Python中,我们可以使用requests库来发送POST请求,并且传入application/x-www-form-urlencoded格式的参数。具体的方法如下:
1. 导入requests库:首先,我们需要导入requests库,因为它提供了简单明了的API来发送HTTP请求。
```
import requests
```
2. 准备参数:我们需要准备指定的参数数据,这些数据需要以字典的形式存储。例如,对于以下的表单数据:
```
username: alice
password: 123456
```
我们可以这样定义字典:
```
payload = {'username': 'alice', 'password': '123456'}
```
3. 发送POST请求:使用requests库的post方法,将参数payload传入,同时设置参数headers中的Content-Type属性为application/x-www-form-urlencoded,以指定发送的是表单数据格式。
```
response = requests.post('http://example.com/login', data=payload, headers={'Content-Type': 'application/x-www-form-urlencoded'})
```
通过这样的HTTP POST请求,我们可以将表单数据payload发送到指定的URL,并且在参数中明确指定了发送的数据格式。在实际的应用中,我们可能还需要处理服务器返回的结果,比如获取响应码、响应头、响应体等信息。我们可以通过使用requests库提供的API来完成这些操作。总之,使用requests库很容易实现POST请求,传递表单数据也非常方便。
相关推荐
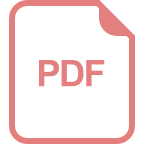
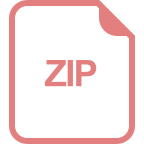
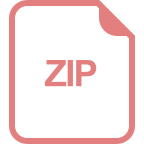














