带约束函数的多目标灰狼算法程序
时间: 2023-11-01 17:11:21 浏览: 119
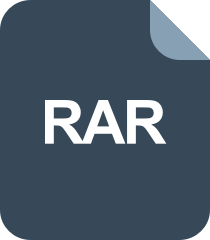
多目标灰狼优化算法MOGWO的MATLAB仿真,matlab2021a测试
以下是带约束函数的多目标灰狼算法程序。该程序采用了一个简单的约束处理方法,即将超出约束范围的解向最近的约束边界移动。
```python
import numpy as np
# 定义目标函数
def objective_function(x):
f1 = x[0] ** 2 + x[1] ** 2
f2 = (x[0] - 1) ** 2 + x[1] ** 2
return [f1, f2]
# 定义约束函数
def constraint_function(x):
g1 = x[0] + x[1] - 2
g2 = -x[0] + x[1] - 2
return [g1, g2]
# 定义灰狼算法
def grey_wolf_optimizer(obj_func, constr_func, lb, ub, dim, n, max_iter):
alpha = np.zeros(dim)
beta = np.zeros(dim)
delta = np.zeros(dim)
alpha_score = float("inf")
beta_score = float("inf")
delta_score = float("inf")
best_scores = []
population = np.zeros((n, dim))
for i in range(n):
population[i, :] = np.random.uniform(lb, ub, dim)
for iter in range(max_iter):
for i in range(n):
# 计算适应度
fitness = obj_func(population[i, :])
# 更新 alpha、beta、delta
if fitness[0] < alpha_score:
alpha_score = fitness[0]
alpha = population[i, :]
if fitness[0] > alpha_score and fitness[0] < beta_score:
beta_score = fitness[0]
beta = population[i, :]
if fitness[0] > alpha_score and fitness[0] > beta_score and fitness[0] < delta_score:
delta_score = fitness[0]
delta = population[i, :]
a = 2 - iter * ((2) / max_iter)
for i in range(n):
# 更新位置
r1 = np.random.random(dim)
r2 = np.random.random(dim)
A1 = 2 * a * r1 - a
C1 = 2 * r2
D_alpha = abs(C1 * alpha - population[i, :])
X1 = alpha - A1 * D_alpha
r1 = np.random.random(dim)
r2 = np.random.random(dim)
A2 = 2 * a * r1 - a
C2 = 2 * r2
D_beta = abs(C2 * beta - population[i, :])
X2 = beta - A2 * D_beta
r1 = np.random.random(dim)
r2 = np.random.random(dim)
A3 = 2 * a * r1 - a
C3 = 2 * r2
D_delta = abs(C3 * delta - population[i, :])
X3 = delta - A3 * D_delta
# 合并个体和三个最优解
new_population = np.vstack([population[i, :], X1, X2, X3])
# 处理约束条件
for j in range(new_population.shape[0]):
for k in range(dim):
if new_population[j, k] < lb[k]:
new_population[j, k] = lb[k]
if new_population[j, k] > ub[k]:
new_population[j, k] = ub[k]
constr_violation = constr_func(new_population[j, :])
if any(constr_violation > 0):
for l in range(len(constr_violation)):
if constr_violation[l] > 0:
new_population[j, :] = np.clip(new_population[j, :], lb, ub)
# 选择新的种群
fitness = np.zeros(new_population.shape[0])
for j in range(new_population.shape[0]):
fitness[j] = obj_func(new_population[j, :])[0]
idx = np.argsort(fitness)
population[i, :] = new_population[idx[0], :]
best_scores.append(alpha_score)
print("Iteration:", iter + 1, " Best Score:", alpha_score)
return alpha, alpha_score, best_scores
# 测试算法
if __name__ == "__main__":
lb = [-4, -4]
ub = [4, 4]
dim = 2
n = 50
max_iter = 100
alpha, alpha_score, best_scores = grey_wolf_optimizer(objective_function, constraint_function, lb, ub, dim, n, max_iter)
print("Best Solution:", alpha)
print("Best Score:", alpha_score)
```
该程序使用了一个列表 `lb` 和 `ub` 来存储每个变量的下限和上限。 `dim` 是变量的数量, `n` 是种群大小, `max_iter` 是算法的最大迭代次数。 程序输出每次迭代的最佳得分和当前迭代次数。 最终,程序输出找到的最佳解以及对应的最佳得分。
阅读全文
相关推荐
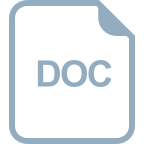
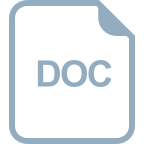
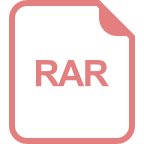
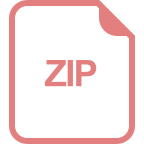
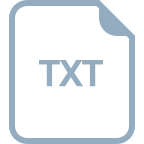
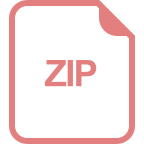
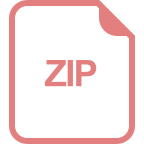
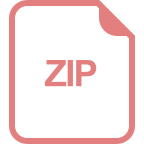
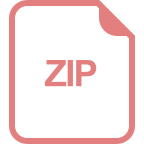
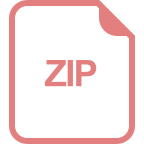
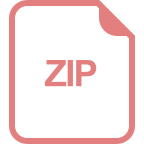
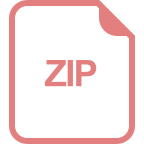
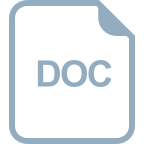
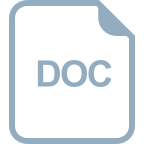
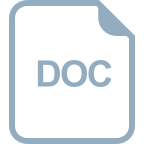
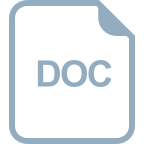

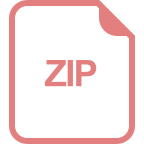