用python写一个扫雷游戏的代码
时间: 2024-12-20 19:32:08 浏览: 6
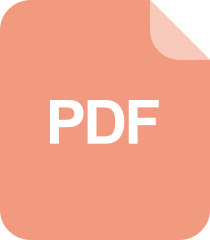
用python写扫雷游戏实例代码分享

编写一个简单的扫雷游戏的Python代码需要用到pygame库来处理图形界面。下面是一个基础版的扫雷游戏的简单框架:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置窗口大小
win_size = (400, 400)
screen = pygame.display.set_mode(win_size)
pygame.display.set_caption("扫雷游戏")
# 定义方块尺寸和数量
cell_size = 50
mine_count = 9
# 创建一个网格列表
grid = [[0 for _ in range((win_size[0] // cell_size) + 1)] for _ in range((win_size[1] // cell_size) + 1)]
mines = random.sample(range(len(grid)**2), mine_count)
# 将随机位置设为地雷
for mine in mines:
x, y = mine // len(grid), mine % len(grid)
grid[x][y] = -1
def draw_grid():
for row in grid:
for cell in row:
if cell == -1:
color = (255, 0, 0) # 红色代表地雷
else:
color = (0, 255, 0) # 绿色代表未发现的地雷
pygame.draw.rect(screen, color, (cell * cell_size, 0, cell_size, cell_size))
# 游戏主循环
running = True
while running:
screen.fill((255, 255, 255)) # 清除屏幕
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONDOWN:
mouse_pos = pygame.mouse.get_pos()
x, y = mouse_pos[0] // cell_size, mouse_pos[1] // cell_size
click_cell(grid, x, y)
draw_grid()
pygame.display.flip()
def click_cell(grid, x, y):
if grid[x][y] == -1: # 如果点击的是地雷
print("Game Over! You stepped on a mine.")
running = False
else:
grid[x][y] += 1 # 该位置周围的地雷数+1
if all(cell >= 0 for row in grid[x:y+1] for cell in row[x:x+1]): # 检查周围是否有地雷
uncover_neighbors(grid, x, y) # 展示周围的格子
def uncover_neighbors(grid, x, y):
# 递归遍历并显示邻居的值
for dx, dy in [(0, -1), (-1, 0), (0, 1), (1, 0)]:
nx, ny = x + dx, y + dy
if 0 <= nx < len(grid) and 0 <= ny < len(grid[0]) and grid[nx][ny] > 0:
uncover_neighbors(grid, nx, ny)
draw_square(nx, ny, grid[nx][ny])
uncover_neighbors(grid, 0, 0) # 开始时揭露左上角的格子
# 游戏结束时退出
pygame.quit()
```
这只是一个基本版本,实际游戏中还需要添加计时、胜利条件、提示功能等。运行这个代码前请确保已经安装了pygame库。
阅读全文
相关推荐
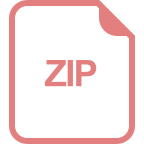
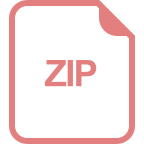





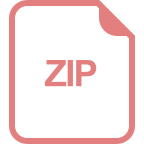
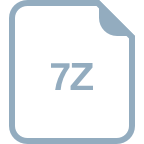