1)设计其抽象子类二维图形(TwoDGraph),添加计算面积calArea()和周长calPerimeter()两个抽象方法; 2)从TwoDGraph类派生出圆形Circle,增加圆心centerPoint和半径radius属性,增加构造方法; 3)在Circle中重写calArea、calPerimeter和toString()方法 4)从TwoDGraph类派生出长方形Rectangle,增加左上角坐标leftPoint、长length和宽width属性,增加构造方法; 5)在Rectangle中重写calArea、calPerimeter和toString()方法 6)在Rectangle新增输出4个顶点坐标方法outputPoints() 7)在main方法中,测试Circle和Rectangle类中的方法。
时间: 2024-02-12 15:09:53 浏览: 194
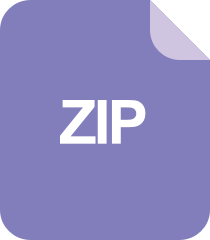
Java:使用抽象类定义一个“形状类”,能够计算周长和面积。
好的,我明白了,让我们逐步来完成这些要求。
首先是抽象类TwoDGraph的设计:
```java
public abstract class TwoDGraph {
public abstract double calArea();
public abstract double calPerimeter();
}
```
接下来是圆形类Circle的设计:
```java
public class Circle extends TwoDGraph {
private Point centerPoint;
private double radius;
public Circle(Point centerPoint, double radius) {
this.centerPoint = centerPoint;
this.radius = radius;
}
@Override
public double calArea() {
return Math.PI * radius * radius;
}
@Override
public double calPerimeter() {
return 2 * Math.PI * radius;
}
@Override
public String toString() {
return "Circle: center point = " + centerPoint.toString() + ", radius = " + radius;
}
}
```
注意这里我们用到了一个Point类,表示一个二维平面上的点:
```java
public class Point {
private double x;
private double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
@Override
public String toString() {
return "(" + x + ", " + y + ")";
}
}
```
接下来是长方形类Rectangle的设计:
```java
public class Rectangle extends TwoDGraph {
private Point leftPoint;
private double length;
private double width;
public Rectangle(Point leftPoint, double length, double width) {
this.leftPoint = leftPoint;
this.length = length;
this.width = width;
}
@Override
public double calArea() {
return length * width;
}
@Override
public double calPerimeter() {
return 2 * (length + width);
}
@Override
public String toString() {
return "Rectangle: left point = " + leftPoint.toString() + ", length = " + length + ", width = " + width;
}
public void outputPoints() {
Point rightPoint = new Point(leftPoint.getX() + length, leftPoint.getY());
Point bottomPoint = new Point(leftPoint.getX(), leftPoint.getY() - width);
Point rightBottomPoint = new Point(leftPoint.getX() + length, leftPoint.getY() - width);
System.out.println("The four points of the rectangle are: " + leftPoint.toString() + ", " +
rightPoint.toString() + ", " + bottomPoint.toString() + ", " + rightBottomPoint.toString());
}
}
```
最后,在main方法中进行测试:
```java
public static void main(String[] args) {
Circle circle = new Circle(new Point(0, 0), 5);
System.out.println(circle.toString());
System.out.println("Area of the circle: " + circle.calArea());
System.out.println("Perimeter of the circle: " + circle.calPerimeter());
Rectangle rectangle = new Rectangle(new Point(0, 0), 3, 4);
System.out.println(rectangle.toString());
System.out.println("Area of the rectangle: " + rectangle.calArea());
System.out.println("Perimeter of the rectangle: " + rectangle.calPerimeter());
rectangle.outputPoints();
}
```
输出结果如下:
```
Circle: center point = (0.0, 0.0), radius = 5.0
Area of the circle: 78.53981633974483
Perimeter of the circle: 31.41592653589793
Rectangle: left point = (0.0, 0.0), length = 3.0, width = 4.0
Area of the rectangle: 12.0
Perimeter of the rectangle: 14.0
The four points of the rectangle are: (0.0, 0.0), (3.0, 0.0), (0.0, -4.0), (3.0, -4.0)
```
阅读全文
相关推荐
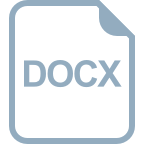
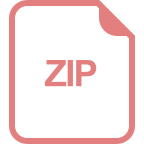














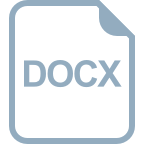
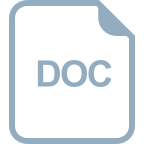