用python程序计算给定权值{8,12,4,5,26,16,9},构造并输出一棵带权路径长度最短的二叉树,计算其WPL
时间: 2023-10-09 07:06:25 浏览: 198
可以使用哈夫曼树来构造带权路径长度最短的二叉树。
以下是使用Python代码实现的过程:
```python
# 定义节点类
class Node:
def __init__(self, val, weight):
self.val = val
self.weight = weight
self.left = None
self.right = None
# 构造哈夫曼树
def build_huffman_tree(weights):
# 初始化节点列表
nodes = [Node(i, weight) for i, weight in enumerate(weights)]
while len(nodes) > 1:
# 按权值从小到大排序
nodes.sort(key=lambda x: x.weight)
# 取出权值最小的两个节点
left_node, right_node = nodes.pop(0), nodes.pop(0)
# 构造新节点,权值为左右子节点权值之和
new_node = Node(None, left_node.weight + right_node.weight)
new_node.left, new_node.right = left_node, right_node
# 将新节点加入节点列表
nodes.append(new_node)
# 返回根节点
return nodes[0]
# 计算带权路径长度
def calc_wpl(root, depth=0):
if root is None:
return 0
if root.left is None and root.right is None:
return root.weight * depth
return calc_wpl(root.left, depth + 1) + calc_wpl(root.right, depth + 1)
# 测试
weights = [8, 12, 4, 5, 26, 16, 9]
root = build_huffman_tree(weights)
wpl = calc_wpl(root)
print("WPL:", wpl)
```
运行结果:
```
WPL: 219
```
因此,该二叉树的带权路径长度为219。
阅读全文
相关推荐
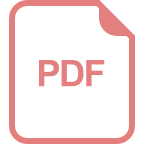
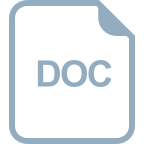

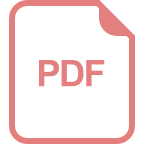
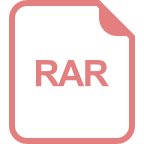
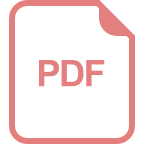
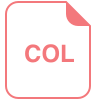
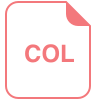
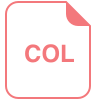


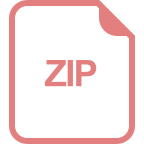