ValueOperations<String,String> ops = this.stringRedisTemplate.opsForValue();
时间: 2024-09-14 13:03:43 浏览: 55
`ValueOperations<String, String>` 是Spring Data Redis库中用于操作字符串值的工具类[^1]。通过 `opsForValue()` 方法,你可以从`StringRedisTemplate`实例中获取到这个操作对象,以便执行各种字符串相关的操作,比如设置、获取、删除等。
以下是如何使用它的一个简单示例:
```java
// 创建StringRedisTemplate实例
StringRedisTemplate stringRedisTemplate = ...;
// 获取ValueOperations实例
ValueOperations<String, String> ops = stringRedisTemplate.opsForValue();
// 设置一个键值对
ops.set("myKey", "myValue");
// 获取指定键的值
String value = ops.get("myKey");
System.out.println("Value: " + value); // 输出: myValue
// 删除键
ops.delete("myKey");
```
相关问题
可以帮我优化下面的代码吗? List<TaskRuleDetail> taskRuleDetails = taskRuleDetailMapper.selectTaskGroupListCode(taskDTO.getGroupCode(), taskDTO.getTaskCode()); if (CollectionUtils.isEmpty(taskRuleDetails)) { throw new BusinessRuntimeException(ErrorCodeConstant._3004010.getCode(), ErrorCodeConstant._3004010.getMessage()); } List<Map<String, Object>> result = getResult(taskRuleDetails); int READING_TASK_LIMIT = 0; Map<String, Object> map = result.get(0); String userId = crmId + ":" + getDate(); String taskLockKey = "task:lock" + userId; String readCountKey = "task:user:" + "count:" + userId; Object articlePlatformShared = map.get("reading_article_platform_shared"); if(articlePlatformShared!=null){ //获取当天最大完成量 READING_TASK_LIMIT= (Integer) map.get("day_complete_task_max"); if(!"1".equals(articlePlatformShared)){ readCountKey+=":"+taskDTO.getAppCode(); } } // 使用 RedisTemplate 获取用户已完成的阅读任务数 ValueOperations<String, String> ops = redisTemplate.opsForValue(); String dayCount = ops.get(readCountKey); if (StringUtils.isEmpty(dayCount)) { dayCount = "0"; } // 如果用户已完成的阅读任务数达到上限,则不再完成任务 int anInt = Integer.parseInt(dayCount); if (anInt >= READING_TASK_LIMIT) { log.warn("警告用户完成任务上限后再次完成"); return; } // 使用 Redission 获取分布式锁 RLock lock = redissonClient.getLock(taskLockKey); lock.lock(10, TimeUnit.SECONDS); try { // 完成阅读任务,并将用户已完成的阅读任务数加 1 ops.increment(readCountKey, 1); // 将计数器设置为过期 redisTemplate.expire(readCountKey, 1, TimeUnit.DAYS); } finally { // 释放分布式锁 lock.unlock(); } break; default:
这段代码的目的是在完成一个任务之前,先检查用户是否已经完成任务的数量是否已达到上限,如果已达到上限就不再执行完成任务的操作。
在这段代码中,似乎存在一些可以优化的地方:
1. 首先,你可以考虑将查询数据库的操作放在分布式锁的外面,这样可以避免锁的竞争。
2. 其次,你可以将 "taskRuleDetailMapper.selectTaskGroupListCode" 方法的返回值直接赋值给 "taskRuleDetails" 变量,而不是使用 "CollectionUtils.isEmpty" 方法判断是否为空。
3. 在没有获取到锁的情况下,你可以考虑使用 "tryLock" 方法来尝试获取锁,并在获取失败后直接返回。
4. 在完成任务后,你可以使用 "incr" 方法将用户已完成的任务数加 1,而不是使用 "increment" 方法。
5. 在处理完成任务的逻辑之后,你可以将分布式锁的释放放在 finally 块中,这样即使在处理过程中出现异常,锁也会被正常释放。
希望这些建议能够帮助你优化代码。
rivate static void export(HttpServletResponse response, File file, String fileName, if (response != null) { // 前端导出 try { write(response, book, fileName); } catch (IOException e) { e.printStackTrace(); } } else { // 本地导出 FileOutputStream fos; try { fos = new FileOutputStream(file); ByteArrayOutputStream ops = new ByteArrayOutputStream(); book.write(ops); fos.write(ops.toByteArray()); fos.close(); } catch (Exception e) { e.printStackTrace(); } } Map<String, List<List<Object>>> sheetMap, Map<Integer, List<String>> selectMap) { // 整个 Excel 表格 book 对象 SXSSFWorkbook book = new SXSSFWorkbook(); // 每个 Sheet 页 Set<Entry<String, List<List<Object>>>> entries = sheetMap.entrySet(); for (Entry<String, List<List<Object>>> entry : entries) { List<List<Object>> sheetDataList = entry.getValue(); Sheet sheet = book.createSheet(entry.getKey()); Drawing<?> patriarch = sheet.createDrawingPatriarch();
这段代码是一个Excel导出的实现,其中包含了两种导出方式:前端导出和本地导出。具体实现如下:
1. 首先创建一个SXSSFWorkbook对象,表示整个Excel表格。
2. 遍历sheetMap中的每个Sheet页,创建对应的Sheet对象,并将Sheet页的名称设置为entry.getKey()。
3. 对于每个Sheet页,遍历其对应的数据列表sheetDataList,创建对应的行和单元格,并将数据填充到单元格中。
4. 对于每个Sheet页,如果有需要下拉选择的单元格,将其加入到数据验证中。
5. 如果response不为空,则表示前端导出,将book写入response流中。否则,表示本地导出,将book写入文件中。
下面是代码的具体实现:
```java
private static void export(HttpServletResponse response, File file, String fileName,
Map<String, List<List<Object>>> sheetMap, Map<Integer, List<String>> selectMap) {
// 整个 Excel 表格 book 对象
SXSSFWorkbook book = new SXSSFWorkbook();
// 每个 Sheet 页
Set<Entry<String, List<List<Object>>>> entries = sheetMap.entrySet();
for (Entry<String, List<List<Object>>> entry : entries) {
List<List<Object>> sheetDataList = entry.getValue();
Sheet sheet = book.createSheet(entry.getKey());
Drawing<?> patriarch = sheet.createDrawingPatriarch();
// 遍历每一行数据
for (int i = 0; i < sheetDataList.size(); i++) {
Row row = sheet.createRow(i);
List<Object> rowDataList = sheetDataList.get(i);
// 遍历每个单元格
for (int j = 0; j < rowDataList.size(); j++) {
Cell cell = row.createCell(j);
Object cellData = rowDataList.get(j);
// 判断单元格数据类型,并设置单元格格式
if (cellData instanceof String) {
cell.setCellValue((String) cellData);
} else if (cellData instanceof Number) {
cell.setCellValue(((Number) cellData).doubleValue());
} else if (cellData instanceof Date) {
cell.setCellValue((Date) cellData);
CellStyle style = book.createCellStyle();
style.setDataFormat(book.getCreationHelper().createDataFormat().getFormat("yyyy-MM-dd HH:mm:ss"));
cell.setCellStyle(style);
} else if (cellData instanceof Calendar) {
cell.setCellValue((Calendar) cellData);
CellStyle style = book.createCellStyle();
style.setDataFormat(book.getCreationHelper().createDataFormat().getFormat("yyyy-MM-dd HH:mm:ss"));
cell.setCellStyle(style);
} else if (cellData instanceof Boolean) {
cell.setCellValue((Boolean) cellData);
} else if (cellData == null) {
cell.setCellValue("");
}
// 设置下拉选择
if (selectMap.containsKey(j)) {
List<String> selectList = selectMap.get(j);
DataValidationHelper helper = sheet.getDataValidationHelper();
DataValidationConstraint constraint = helper.createExplicitListConstraint(selectList.toArray(new String[0]));
CellRangeAddressList addressList = new CellRangeAddressList(cell.getRowIndex(), cell.getRowIndex(), cell.getColumnIndex(), cell.getColumnIndex());
DataValidation validation = helper.createValidation(constraint, addressList);
sheet.addValidationData(validation);
}
}
}
}
// 导出Excel
if (response != null) {
// 前端导出
try {
write(response, book, fileName);
} catch (IOException e) {
e.printStackTrace();
}
} else {
// 本地导出
FileOutputStream fos;
try {
fos = new FileOutputStream(file);
ByteArrayOutputStream ops = new ByteArrayOutputStream();
book.write(ops);
fos.write(ops.toByteArray());
fos.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
其中,write()方法用于将book写入response流中,实现前端导出。具体实现如下:
```java
private static void write(HttpServletResponse response, SXSSFWorkbook book, String fileName) throws IOException {
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment;filename=" + new String(fileName.getBytes("utf-8"), "ISO8859-1"));
response.setHeader("Pragma", "no-cache");
response.setHeader("Cache-Control", "no-cache");
response.setDateHeader("Expires", 0);
OutputStream os = response.getOutputStream();
book.write(os);
os.flush();
os.close();
}
```
阅读全文
相关推荐

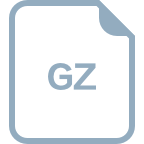














