paddle.layer转化成torch
时间: 2023-07-03 15:14:37 浏览: 54
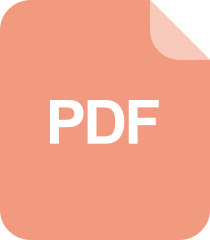
问题解决:AttributeError: module ‘paddle.fluid’ has no attribute ‘EndStepEvent’

To convert a neural network defined using `paddle.layer` to PyTorch, you would need to manually translate the layer definitions, parameter initializations, and computation graph definition. Here's an example of how to translate a simple neural network defined using `paddle.layer` to PyTorch:
```
import paddle.fluid as fluid
import paddle.fluid.layers as layers
import torch.nn as nn
import torch.nn.functional as F
# Define a simple neural network using paddle.layer
x = layers.data(name='x', shape=[784], dtype='float32')
hidden = layers.fc(input=x, size=256, act='relu')
output = layers.fc(input=hidden, size=10, act=None)
# Translate the network to PyTorch
class MyNet(nn.Module):
def __init__(self):
super(MyNet, self).__init__()
self.linear1 = nn.Linear(784, 256)
self.linear2 = nn.Linear(256, 10)
def forward(self, x):
x = F.relu(self.linear1(x))
x = self.linear2(x)
return x
# Copy the parameter values from paddle.layer to PyTorch
with fluid.dygraph.guard():
param_state_dict, _ = fluid.dygraph.load_dygraph('model')
model = MyNet()
model_dict = model.state_dict()
for k in model_dict.keys():
if k in param_state_dict:
model_dict[k] = torch.from_numpy(param_state_dict[k].numpy())
model.load_state_dict(model_dict)
```
In this example, we define a simple neural network using `paddle.layer`, which consists of two fully-connected layers with ReLU activation and no activation function, respectively. We then manually translate the network to PyTorch by defining a corresponding `nn.Module` subclass with `nn.Linear` layers for the fully-connected layers and `F.relu` activation function. We also use the `fluid.dygraph.load_dygraph` function to load the parameter values from a saved PaddlePaddle model and copy them to the PyTorch model.
Note that this is just an example, and the specific translation process may vary depending on the complexity of the network and the specific modules used in the PaddlePaddle version.
阅读全文
相关推荐
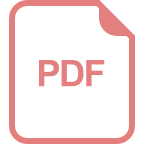
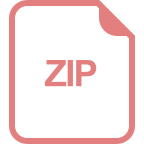
















