读取文件夹中某文档的数据
时间: 2023-11-12 19:08:38 浏览: 39
这个问题需要更具体的信息,因为读取文件夹中某个文档的数据取决于你使用的编程语言和操作系统。以下是一些可能有用的信息:
- Python:你可以使用 `os` 模块中的 `listdir` 函数获取文件夹中的所有文件列表,然后使用 `open` 函数打开指定的文档并读取其中的数据。例如:
```
import os
dir_path = '/path/to/folder'
file_name = 'example.txt'
files = os.listdir(dir_path)
if file_name in files:
file_path = os.path.join(dir_path, file_name)
with open(file_path, 'r') as f:
data = f.read()
print(data)
else:
print('File not found')
```
- Java:你可以使用 `File` 类和 `FileReader` 类来读取文件夹中的文档数据。例如:
```
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class ReadFile {
public static void main(String[] args) throws IOException {
String dirPath = "/path/to/folder";
String fileName = "example.txt";
File dir = new File(dirPath);
if (dir.isDirectory()) {
File file = new File(dir, fileName);
if (file.isFile()) {
FileReader reader = new FileReader(file);
char[] buffer = new char[1024];
int read = reader.read(buffer);
String data = new String(buffer, 0, read);
System.out.println(data);
reader.close();
} else {
System.out.println("File not found");
}
} else {
System.out.println("Folder not found");
}
}
}
```
- C++:你可以使用 `dirent.h` 库来读取文件夹中的文档数据。例如:
```
#include <iostream>
#include <dirent.h>
#include <fstream>
#include <string>
using namespace std;
int main() {
string folder_path = "/path/to/folder";
string file_name = "example.txt";
DIR* dir = opendir(folder_path.c_str());
if (dir) {
dirent* entry;
while ((entry = readdir(dir)) != NULL) {
if (entry->d_name == file_name) {
string file_path = folder_path + "/" + file_name;
ifstream file(file_path);
if (file.is_open()) {
string data((istreambuf_iterator<char>(file)),
istreambuf_iterator<char>());
cout << data << endl;
file.close();
} else {
cout << "File not found" << endl;
}
break;
}
}
closedir(dir);
} else {
cout << "Folder not found" << endl;
}
return 0;
}
```
请注意,这些示例代码仅供参考,你需要根据自己的具体情况进行修改和调整。
相关推荐
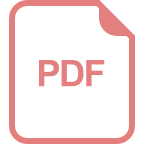
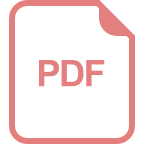














