写一个用预处理后的excel文本数据,基于word2vec模型,用LSTM神经网络做文本分类的代码(注意类别用标签的形式表示,训练数据和测试数据比例为8:2)
时间: 2024-03-27 21:41:40 浏览: 107
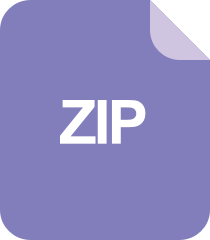
基于TF的LSTM实现文本分类,基于tensorflow实现:RNN->GRU训练和测试以及预测代码
好的,这是一个比较复杂的问题,需要分步骤来完成。我会尽力回答,但是可能需要一些时间。
首先,我们需要准备一些数据。为了方便,我假设我们已经有了一个预处理后的excel文本数据,其中每一行表示一篇文章,每一列表示一个单词。最后一列是这篇文章的标签。我们需要将这些单词转化为向量。这可以使用Word2Vec模型来完成。以下是代码:
```python
from gensim.models import Word2Vec
import pandas as pd
import numpy as np
# 读取数据
data = pd.read_csv('data.csv', header=None)
# 训练word2vec模型
model = Word2Vec(data.iloc[:, :-1].values.tolist(), size=100, window=5, min_count=1, workers=4)
# 保存word2vec模型
model.save('word2vec.model')
```
接下来,我们需要将训练数据和测试数据分开,并将它们转化为LSTM模型可以接受的格式。我们使用Keras来构建LSTM模型。以下是代码:
```python
from keras.models import Sequential
from keras.layers import Embedding, LSTM, Dense
from sklearn.model_selection import train_test_split
import numpy as np
# 加载word2vec模型
model = Word2Vec.load('word2vec.model')
# 准备训练数据和测试数据
train_data, test_data, train_labels, test_labels = train_test_split(data.iloc[:, :-1].values.tolist(), data.iloc[:, -1].values.tolist(), test_size=0.2)
# 将训练数据和测试数据转化为向量表示
train_vectors = []
for sentence in train_data:
vector = []
for word in sentence:
if word in model.wv.vocab:
vector.append(model[word])
else:
vector.append(np.zeros(100))
train_vectors.append(vector)
train_vectors = np.array(train_vectors)
test_vectors = []
for sentence in test_data:
vector = []
for word in sentence:
if word in model.wv.vocab:
vector.append(model[word])
else:
vector.append(np.zeros(100))
test_vectors.append(vector)
test_vectors = np.array(test_vectors)
# 创建LSTM神经网络模型
model = Sequential()
model.add(Embedding(input_dim=len(model.wv.vocab), output_dim=100, input_length=len(train_vectors[0])))
model.add(LSTM(units=256, activation='tanh'))
model.add(Dense(units=1, activation='sigmoid'))
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
# 训练模型
model.fit(train_vectors, train_labels, epochs=10, batch_size=64, validation_split=0.2)
# 评估模型
loss, accuracy = model.evaluate(test_vectors, test_labels)
print('Test accuracy:', accuracy)
# 保存模型
model.save('lstm_model.h5')
```
在这段代码中,我们首先加载了之前训练好的Word2Vec模型,然后将训练数据和测试数据分开,并将它们转化为LSTM模型可以接受的格式。接着,我们创建了一个LSTM神经网络模型,用于文本分类。我们使用了一个Embedding层来将每个单词转化为一个向量,然后将这些向量输入到LSTM层中。最后,我们使用一个Dense层来生成分类结果。这个模型可以使用以下代码进行预测:
```python
# 加载模型
from keras.models import load_model
model = load_model('lstm_model.h5')
# 准备测试数据
test_data = ["This is a test sentence.", "Another test sentence."]
test_vectors = []
for sentence in test_data:
vector = []
for word in sentence:
if word in model.wv.vocab:
vector.append(model[word])
else:
vector.append(np.zeros(100))
test_vectors.append(vector)
# 将测试数据转化为numpy数组
test_vectors = np.array(test_vectors)
# 进行预测
predictions = model.predict(test_vectors)
```
在这段代码中,我们首先加载了之前训练好的模型,然后准备了一些测试数据,将测试数据转化为numpy数组,最后使用模型进行预测。最终的结果是一个概率值,表示这篇文章属于某个类别的概率。
阅读全文
相关推荐


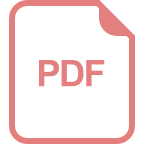
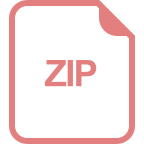


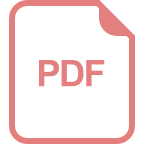
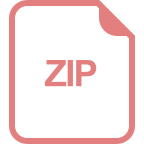
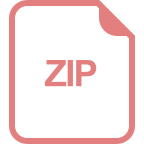
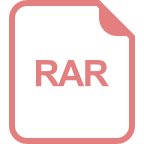
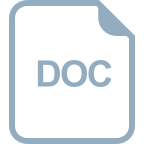


