写一个用excel文本数据,基于word2vec模型,用LSTM神经网络做文本分类的代码(注意类别用标签的形式表示)
时间: 2024-03-27 20:35:55 浏览: 14
好的,以下是一个基于Python和Keras的文本分类代码示例,包括对Excel文本数据进行读取、预处理、Word2Vec向量化、LSTM模型训练和测试:
```python
import pandas as pd
import numpy as np
import nltk
from gensim.models import Word2Vec
from keras.models import Sequential
from keras.layers import LSTM, Dense, Dropout
from keras.preprocessing.text import Tokenizer
from keras.preprocessing.sequence import pad_sequences
# 读取Excel文件
df = pd.read_excel('data.xlsx')
# 数据预处理
nltk.download('stopwords')
stopwords = nltk.corpus.stopwords.words('english')
def preprocess_text(text):
# 分词
tokens = nltk.word_tokenize(text.lower())
# 去除停用词和标点符号
tokens = [token for token in tokens if token not in stopwords and token.isalpha()]
# 连接成字符串
return " ".join(tokens)
df['text'] = df['text'].apply(preprocess_text)
# 训练Word2Vec模型
sentences = [nltk.word_tokenize(text) for text in df['text']]
word2vec = Word2Vec(sentences, size=100, window=5, min_count=1, workers=4)
# 将文本转化为向量表示
tokenizer = Tokenizer()
tokenizer.fit_on_texts(df['text'])
X = tokenizer.texts_to_sequences(df['text'])
X = pad_sequences(X, maxlen=100)
# 将标签转化为向量表示
Y = pd.get_dummies(df['label']).values
# 构建LSTM模型
model = Sequential()
model.add(LSTM(128, dropout=0.2, recurrent_dropout=0.2, input_shape=(100, )))
model.add(Dense(64, activation='relu'))
model.add(Dropout(0.5))
model.add(Dense(Y.shape[1], activation='softmax'))
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
print(model.summary())
# 训练模型
model.fit(X, Y, epochs=10, batch_size=32)
# 测试模型
test_text = "This is a test text"
test_text = preprocess_text(test_text)
test_vector = tokenizer.texts_to_sequences([test_text])
test_vector = pad_sequences(test_vector, maxlen=100)
result = model.predict(test_vector)[0]
label = np.argmax(result)
print("Predicted label:", label)
```
需要注意的是,Excel文本数据的格式应该是一列为文本内容,另一列为文本分类标签。在本示例中,我们使用`pd.get_dummies()`将分类标签转化为了向量表示。
此外,由于Word2Vec模型和LSTM模型的训练需要消耗大量的计算资源,建议在GPU环境下运行代码。如果您没有GPU资源,可以尝试使用预训练的Word2Vec模型和LSTM模型,或者使用更轻量级的文本分类算法。
相关推荐
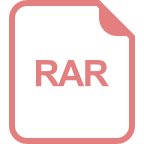














