通过面向对象编程思想使用paramiko中的invoke_shell,一直批量ping数量未知的IP
时间: 2023-11-28 09:50:23 浏览: 140
可以使用以下的代码实现:
```python
import paramiko
class SSHClient:
def __init__(self, host, port, username, password):
self.host = host
self.port = port
self.username = username
self.password = password
self.client = paramiko.SSHClient()
self.client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
self.client.connect(self.host, self.port, self.username, self.password)
def exec_command(self, command):
stdin, stdout, stderr = self.client.exec_command(command)
return stdout.readlines()
def invoke_shell(self):
self.shell = self.client.invoke_shell()
return self.shell
def send_command(self, command):
self.shell.send(command + "\n")
while not self.shell.recv_ready():
pass
output = self.shell.recv(10000).decode('utf-8')
return output
def close(self):
self.client.close()
def ping_ips(ip_list):
ssh = SSHClient('your_server_ip', 22, 'your_username', 'your_password')
shell = ssh.invoke_shell()
shell.recv(1000)
shell.send("sudo su\n")
shell.recv(1000)
shell.send("echo '' > ping_result.txt\n")
shell.recv(1000)
for ip in ip_list:
shell.send("ping -c 1 " + ip + " >> ping_result.txt\n")
shell.recv(1000)
shell.send("exit\n")
ssh.close()
with open('ping_result.txt', 'r') as f:
result = f.read()
return result
```
这个 `SSHClient` 类可以用来连接远程服务器并执行命令。`invoke_shell()` 方法会返回一个交互式的 shell,此时可以输入命令并获取输出。在这个例子中,我们使用 `sudo su` 命令切换到 root 用户,然后使用 `ping` 命令批量 ping IP,并将结果保存到文件中。最后从文件中读取结果并返回。
阅读全文
相关推荐










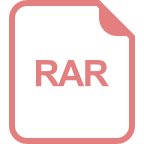

