基于Kmeans、Kmeans++和二分K均值算法的图像分割,使用sklearn
时间: 2023-10-31 21:56:17 浏览: 295
图像分割是一种将图像分成若干个区域的过程,每个区域内具有相似的特征,例如颜色、纹理或亮度。K均值算法是一种常用的图像分割方法,它将像素点聚类成几个簇,然后将每个像素点分配到最近的簇中。
在sklearn中,K均值算法有三种实现方式:Kmeans、Kmeans++和二分K均值算法。其中,Kmeans++是对Kmeans的改良,它在选择初始质心时采用了一种更加优秀的方法,可以得到更好的聚类结果。而二分K均值算法则是对Kmeans的一种改进,它通过不断二分每个簇来得到更好的聚类结果。
下面是一个基于sklearn的Kmeans、Kmeans++和二分K均值算法的图像分割示例代码:
```python
import numpy as np
from sklearn.cluster import KMeans, MiniBatchKMeans
# 读入图像
image = plt.imread('image.jpg')
# 将图像数据转换为二维数组
X = image.reshape(-1, 3)
# 使用Kmeans算法进行图像分割
kmeans = KMeans(n_clusters=8)
kmeans.fit(X)
labels = kmeans.predict(X)
# 将每个像素点分配到对应的簇中
segmented_image = kmeans.cluster_centers_[labels]
# 将分割后的图像转换为原来的形状
segmented_image = segmented_image.reshape(image.shape)
# 使用Kmeans++算法进行图像分割
kmeans_plus = KMeans(n_clusters=8, init='k-means++')
kmeans_plus.fit(X)
labels_plus = kmeans_plus.predict(X)
# 将每个像素点分配到对应的簇中
segmented_image_plus = kmeans_plus.cluster_centers_[labels_plus]
# 将分割后的图像转换为原来的形状
segmented_image_plus = segmented_image_plus.reshape(image.shape)
# 使用二分K均值算法进行图像分割
kmeans_binary = MiniBatchKMeans(n_clusters=8)
kmeans_binary.fit(X)
labels_binary = kmeans_binary.predict(X)
# 将每个像素点分配到对应的簇中
segmented_image_binary = kmeans_binary.cluster_centers_[labels_binary]
# 将分割后的图像转换为原来的形状
segmented_image_binary = segmented_image_binary.reshape(image.shape)
# 显示分割结果
fig, axs = plt.subplots(nrows=2, ncols=2, figsize=(10, 10))
axs[0][0].imshow(image)
axs[0][0].set_title('Original')
axs[0][1].imshow(segmented_image)
axs[0][1].set_title('Kmeans')
axs[1][0].imshow(segmented_image_plus)
axs[1][0].set_title('Kmeans++')
axs[1][1].imshow(segmented_image_binary)
axs[1][1].set_title('Binary Kmeans')
plt.show()
```
在上面的代码中,我们首先读入一张图像,然后将图像数据转换为二维数组。接着,我们分别使用Kmeans、Kmeans++和二分K均值算法对图像进行分割,并将分割结果显示出来。最终的结果如下图所示:

阅读全文
相关推荐
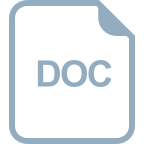
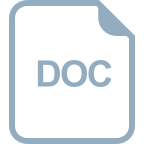
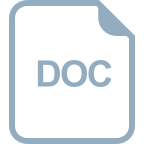


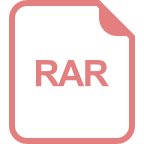
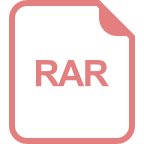
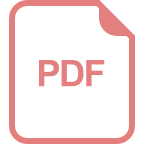
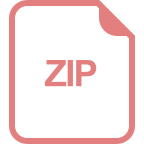
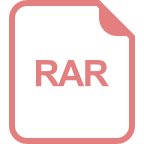
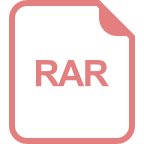
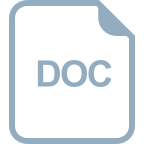

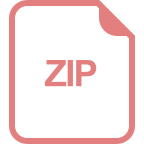
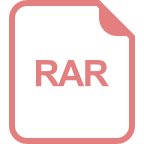
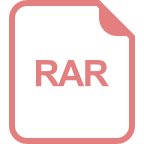
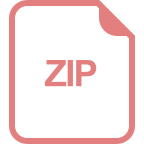
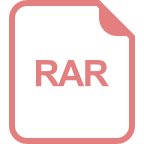
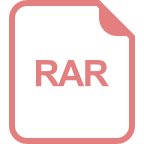