CShap sleep sort睡眠排序 源代码
时间: 2023-10-08 12:08:01 浏览: 89
以下是C#实现的睡眠排序(Sleep Sort)的源代码:
```csharp
using System;
using System.Threading;
class SleepSort {
static void Main(string[] args) {
int[] arr = {5, 3, 9, 4, 1, 6, 8, 2, 7};
foreach (int num in arr) {
new Thread(() => {
Thread.Sleep(num * 1000);
Console.WriteLine(num);
}).Start();
}
}
}
```
该代码首先定义了一个整数数组 `arr`,然后遍历该数组中的每个元素,创建一个新线程并将该元素作为参数传递给线程。在每个线程中,线程将休眠 `num` 秒,然后输出该数字。
由于每个线程的休眠时间不同,因此数字将按升序输出。例如,假设数组中有数字 `5` 和 `3`,则线程 `3` 会在线程 `5` 之前完成,因此 `3` 将先于 `5` 输出。
要使用不同的数字,请修改数组 `arr` 中的元素。请注意,由于该算法涉及线程,因此可能会影响系统性能,因此请谨慎使用。
相关问题
CShap数字字符串排序 源代码
以下是一个用C#编写的数字字符串排序的源代码:
using System;
using System.Linq;
class Program
{
static void Main(string[] args)
{
string[] numbers = { "10", "9", "100", "200", "50" };
Console.WriteLine("排序前:");
foreach (string number in numbers)
{
Console.Write(number + " ");
}
Array.Sort(numbers, (a, b) => int.Parse(a).CompareTo(int.Parse(b)));
Console.WriteLine("\n排序后:");
foreach (string number in numbers)
{
Console.Write(number + " ");
}
Console.ReadLine();
}
}
该程序首先声明一个包含数字字符串的数组,然后使用Array.Sort方法进行排序。在这个例子中,使用lambda表达式作为比较器来确保以数字方式排序。最后,程序在控制台上打印排序前和排序后的数字字符串。
CShap 图的拓扑排序 源代码
下面是C#中实现图的拓扑排序的源代码:
```csharp
using System;
using System.Collections.Generic;
namespace TopologicalSort
{
class Graph
{
private int V; // 顶点的数量
private List<int>[] adj; // 邻接表
public Graph(int v)
{
V = v;
adj = new List<int>[v];
for (int i = 0; i < v; i++)
{
adj[i] = new List<int>();
}
}
// 添加边
public void AddEdge(int v, int w)
{
adj[v].Add(w);
}
// 拓扑排序
public void TopologicalSort()
{
// 统计每个顶点的入度
int[] indegree = new int[V];
for (int i = 0; i < V; i++)
{
List<int> adjList = adj[i];
foreach (int vertex in adjList)
{
indegree[vertex]++;
}
}
// 创建一个队列,用于存储入度为0的顶点
Queue<int> queue = new Queue<int>();
for (int i = 0; i < V; i++)
{
if (indegree[i] == 0)
{
queue.Enqueue(i);
}
}
// 从队列中弹出顶点并输出
int count = 0;
List<int> result = new List<int>();
while (queue.Count > 0)
{
int vertex = queue.Dequeue();
result.Add(vertex);
foreach (int adjVertex in adj[vertex])
{
indegree[adjVertex]--;
if (indegree[adjVertex] == 0)
{
queue.Enqueue(adjVertex);
}
}
count++;
}
// 判断是否存在环
if (count != V)
{
Console.WriteLine("图中存在环!");
}
else
{
// 输出拓扑排序结果
Console.WriteLine("拓扑排序结果:");
foreach (int vertex in result)
{
Console.Write(vertex + " ");
}
}
}
}
class Program
{
static void Main(string[] args)
{
Graph g = new Graph(6);
g.AddEdge(5, 2);
g.AddEdge(5, 0);
g.AddEdge(4, 0);
g.AddEdge(4, 1);
g.AddEdge(2, 3);
g.AddEdge(3, 1);
g.TopologicalSort();
Console.ReadKey();
}
}
}
```
阅读全文
相关推荐
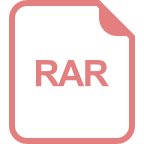












