unity人物旋转脚本代码
时间: 2023-03-12 09:13:59 浏览: 216
基本的人物旋转脚本代码可以通过以下步骤实现:1)使用Unity的transform.Rotate()方法来设置人物的旋转角度。2)使用Input.GetAxis()方法来获取用户输入的角度值。3)使用Vector3.up来设置角度的增量值。4)使用transform.Rotate()方法将角度增量添加到人物的当前角度上。5)重复1-4步骤以实现人物旋转。
相关问题
unity3d 人物 移动脚本
以下是一个简单的Unity3D人物移动脚本:
```csharp
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float speed = 6.0f; // 移动速度
private Rigidbody rb; // 刚体
void Start()
{
rb = GetComponent<Rigidbody>(); // 获取刚体组件
}
void FixedUpdate()
{
float moveHorizontal = Input.GetAxis("Horizontal"); // 获取水平方向输入
float moveVertical = Input.GetAxis("Vertical"); // 获取垂直方向输入
Vector3 movement = new Vector3(moveHorizontal, 0.0f, moveVertical); // 构建移动向量
rb.AddForce(movement * speed); // 添加力以移动角色
}
}
```
这个脚本使用Unity的Rigidbody组件来控制角色移动。在Start函数中,我们获取了角色的刚体组件。在FixedUpdate函数中,我们使用Input.GetAxis函数来获取玩家的水平和垂直输入,并将它们存储在一个Vector3变量movement中。然后,我们使用rb.AddForce函数将movement向量与速度乘积相乘并添加到刚体中,以实现角色的移动。
请注意,此脚本仅控制角色的移动。如果您需要控制其他方面,例如跳跃或旋转,请在脚本中添加额外的代码。
unity实现人物移动旋转代码
以下是一个简单的示例代码,可以实现人物的移动和旋转:
```csharp
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float speed = 5f; // 移动速度
public float rotateSpeed = 100f; // 旋转速度
private Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void FixedUpdate()
{
// 获取水平和垂直输入
float h = Input.GetAxisRaw("Horizontal");
float v = Input.GetAxisRaw("Vertical");
// 计算移动方向和速度
Vector3 moveDir = new Vector3(h, 0f, v).normalized;
Vector3 moveVel = moveDir * speed;
// 应用移动力
rb.velocity = moveVel;
// 如果有输入则旋转角色
if (moveDir != Vector3.zero)
{
Quaternion targetRotation = Quaternion.LookRotation(moveDir);
rb.MoveRotation(Quaternion.RotateTowards(transform.rotation, targetRotation, rotateSpeed * Time.fixedDeltaTime));
}
}
}
```
说明:
- 该脚本需要挂载在角色对象上。
- `speed` 和 `rotateSpeed` 分别控制角色的移动速度和旋转速度。
- 在 `Start` 方法中获取角色的 `Rigidbody` 组件。
- 在 `FixedUpdate` 方法中获取水平和垂直输入,并计算出移动方向和速度。
- 将移动速度应用到角色的 `Rigidbody` 组件上。
- 如果有输入,则将角色的旋转角度逐渐调整到目标旋转角度,以实现平滑的旋转。
阅读全文
相关推荐
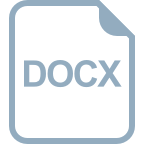
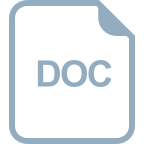








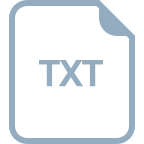
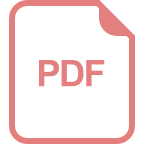

