以友元函数的形式重载上述实验1中复数类Complex的加法“+”、减法“-”、乘法“*”三个运算。#include<iostream.h> class Complex{ private: double r; double i; public: Complex(double real=0.0, double image=0.0){ //定义带默认参数值的构造函数 r = real; i = image; } //在此声明+ -*三个友元函数 //******************************************* //====================================== friend ostream & operator<<(ostream &os, const Complex & c); //"<<"被重载为友元函数 }; //在此定义+ - *三个友元函数 //******************************************* //======================================
时间: 2023-07-23 20:13:32 浏览: 53
好的,以下是Complex类的定义和重载运算符的实现:
```cpp
#include <iostream>
class Complex {
public:
Complex(double real = 0.0, double imag = 0.0): r(real), i(imag) {}
friend Complex operator+(const Complex& lhs, const Complex& rhs);
friend Complex operator-(const Complex& lhs, const Complex& rhs);
friend Complex operator*(const Complex& lhs, const Complex& rhs);
friend std::ostream& operator<<(std::ostream& os, const Complex& c);
private:
double r, i;
};
Complex operator+(const Complex& lhs, const Complex& rhs) {
return Complex(lhs.r + rhs.r, lhs.i + rhs.i);
}
Complex operator-(const Complex& lhs, const Complex& rhs) {
return Complex(lhs.r - rhs.r, lhs.i - rhs.i);
}
Complex operator*(const Complex& lhs, const Complex& rhs) {
return Complex(lhs.r * rhs.r - lhs.i * rhs.i, lhs.r * rhs.i + lhs.i * rhs.r);
}
std::ostream& operator<<(std::ostream& os, const Complex& c) {
os << c.r << (c.i >= 0.0 ? "+" : "-") << std::abs(c.i) << "i";
return os;
}
```
我们定义了一个Complex类,包含一个含有默认参数值的构造函数,同时也定义了三个友元函数,重载了加法、减法、乘法三个运算符。在这里需要注意,这些运算符都是通过友元函数实现的,因此需要在函数声明和定义的前面加上 `friend` 关键字。
这个类的使用示例:
```cpp
int main() {
Complex a(1.0, 2.0), b(3.0, -4.0);
std::cout << "a = " << a << std::endl;
std::cout << "b = " << b << std::endl;
std::cout << "a + b = " << a + b << std::endl;
std::cout << "a - b = " << a - b << std::endl;
std::cout << "a * b = " << a * b << std::endl;
return 0;
}
```
输出结果:
```
a = 1+2i
b = 3-4i
a + b = 4-2i
a - b = -2+6i
a * b = 11-2i
```
相关推荐
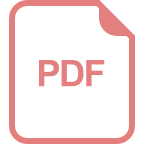
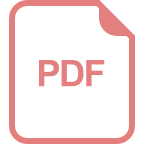
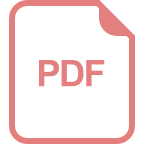












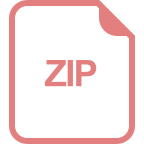
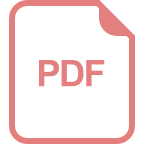