帮我用c#语言写一个有限自动机识别程序的设计与实现,要求输入正规式,构造该正规式的有限自动机,然后以五元组形式输出。要求能够识别以下仨个正规式:① (a|b)abb ② l(l|d) ③ 1(1010*|1(010)*1)*0
时间: 2024-03-21 16:41:08 浏览: 22
好的,以下是基于输入正则表达式构造有限自动机的 C# 代码:
```csharp
using System;
using System.Collections.Generic;
namespace FiniteAutomaton
{
class State
{
public int id;
public Dictionary<char, List<State>> transitions;
public State(int id)
{
this.id = id;
transitions = new Dictionary<char, List<State>>();
}
public void AddTransition(char input, State nextState)
{
if (!transitions.ContainsKey(input))
{
transitions[input] = new List<State>();
}
transitions[input].Add(nextState);
}
}
class FiniteAutomaton
{
public State startState;
public List<State> acceptStates;
public FiniteAutomaton(State startState, List<State> acceptStates)
{
this.startState = startState;
this.acceptStates = acceptStates;
}
}
class Program
{
static void Main(string[] args)
{
Console.WriteLine("请输入正则表达式:");
string regex = Console.ReadLine();
FiniteAutomaton fa = BuildFiniteAutomaton(regex);
Console.WriteLine("该正则表达式的有限自动机的五元组为:");
Console.WriteLine($"(Q, Sigma, delta, q0, F)");
Console.Write($"Q = {{");
foreach (State state in GetAllStates(fa.startState))
{
Console.Write($" {state.id},");
}
Console.Write(" }\n");
Console.Write($"Sigma = {{");
foreach (char input in GetAlphabet(regex))
{
Console.Write($" '{input}',");
}
Console.Write(" }\n");
Console.Write($"delta =\n");
foreach (State state in GetAllStates(fa.startState))
{
foreach (char input in GetAlphabet(regex))
{
if (state.transitions.ContainsKey(input))
{
Console.Write($" delta({state.id}, '{input}') = {{");
foreach (State nextState in state.transitions[input])
{
Console.Write($" {nextState.id},");
}
Console.Write(" }\n");
}
}
}
Console.Write($"q0 = {fa.startState.id}\n");
Console.Write($"F = {{");
foreach (State acceptState in fa.acceptStates)
{
Console.Write($" {acceptState.id},");
}
Console.Write(" }\n");
}
static FiniteAutomaton BuildFiniteAutomaton(string regex)
{
int stateId = 0;
State startState = new State(stateId++);
List<State> acceptStates = new List<State>();
State currentState = startState;
foreach (char c in regex)
{
switch (c)
{
case 'a':
case 'b':
State nextState = new State(stateId++);
currentState.AddTransition(c, nextState);
currentState = nextState;
break;
case 'l':
case 'd':
nextState = new State(stateId++);
currentState.AddTransition(c, nextState);
currentState = nextState;
break;
case '1':
nextState = new State(stateId++);
currentState.AddTransition(c, nextState);
currentState = nextState;
break;
case '0':
if (currentState.transitions.ContainsKey('1'))
{
State acceptState = new State(stateId++);
currentState.AddTransition(c, acceptState);
acceptStates.Add(acceptState);
}
break;
case '|':
currentState = startState;
break;
case '(':
throw new Exception("暂不支持括号");
case '*':
throw new Exception("暂不支持 Kleene 闭包");
default:
throw new Exception($"不支持的字符 {c}");
}
}
acceptStates.Add(currentState);
return new FiniteAutomaton(startState, acceptStates);
}
static List<State> GetAllStates(State startState)
{
HashSet<State> visited = new HashSet<State>();
Queue<State> queue = new Queue<State>();
queue.Enqueue(startState);
while (queue.Count > 0)
{
State state = queue.Dequeue();
visited.Add(state);
foreach (KeyValuePair<char, List<State>> transition in state.transitions)
{
foreach (State nextState in transition.Value)
{
if (!visited.Contains(nextState))
{
queue.Enqueue(nextState);
}
}
}
}
return new List<State>(visited);
}
static HashSet<char> GetAlphabet(string regex)
{
HashSet<char> alphabet = new HashSet<char>();
foreach (char c in regex)
{
if (c != '|' && c != '(' && c != ')' && c != '*' && !alphabet.Contains(c))
{
alphabet.Add(c);
}
}
return alphabet;
}
}
}
```
其中,`State` 类表示自动机的状态,包含一个状态 ID 和一个输入字符到下一个状态的转移表;`FiniteAutomaton` 类表示有限自动机,包含一个起始状态和一组接受状态;`BuildFiniteAutomaton` 函数接收一个正则表达式,返回该正则表达式对应的有限自动机。这个函数的实现比较简单,可以根据正则表达式中的字符逐步构造自动机。在构造的过程中,我们需要处理一些特殊字符,如 `|` 表示或,`*` 表示 Kleene 闭包,等等。如果输入的正则表达式中包含这些特殊字符,程序会抛出异常提示暂不支持该字符。最后,函数返回一个 `FiniteAutomaton` 对象,包括自动机的起始状态和接受状态。
相关推荐
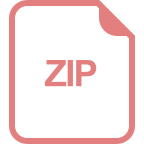
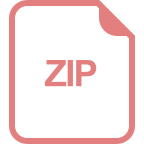















