请用c语言实现对两个升序链表的合并
时间: 2023-09-07 13:14:29 浏览: 44
好的,以下是C语言实现对两个升序链表的合并的代码:
```c
#include <stdio.h>
#include <stdlib.h>
/* 定义链表节点结构体 */
struct Node {
int data;
struct Node* next;
};
/* 链表节点插入函数 */
void push(struct Node** head_ref, int new_data)
{
/* 为新的节点分配内存 */
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
/* 将数据赋值给新的节点 */
new_node->data = new_data;
/* 将新节点的下一个节点指向当前头结点 */
new_node->next = (*head_ref);
/* 将头结点指向新节点 */
(*head_ref) = new_node;
}
/* 合并两个升序链表的函数 */
struct Node* mergeLists(struct Node* a, struct Node* b)
{
struct Node* result = NULL;
/* 如果其中一个链表为空,则直接返回另一个链表 */
if (a == NULL)
return (b);
else if (b == NULL)
return (a);
/* 递归比较链表中的每个节点,将较小的节点插入到result链表中 */
if (a->data <= b->data) {
result = a;
result->next = mergeLists(a->next, b);
}
else {
result = b;
result->next = mergeLists(a, b->next);
}
return (result);
}
/* 输出链表的函数 */
void printList(struct Node* node)
{
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
}
/* 主函数 */
int main()
{
struct Node* res = NULL;
/* 创建两个升序链表 */
struct Node* a = NULL;
struct Node* b = NULL;
push(&a, 15);
push(&a, 10);
push(&a, 5);
push(&b, 20);
push(&b, 3);
push(&b, 2);
/* 输出两个升序链表 */
printf("List 1: \n");
printList(a);
printf("\n");
printf("List 2: \n");
printList(b);
printf("\n");
/* 合并两个升序链表 */
res = mergeLists(a, b);
/* 输出合并后的链表 */
printf("Merged list: \n");
printList(res);
printf("\n");
return 0;
}
```
希望这个代码可以帮助解决你的问题。
相关推荐
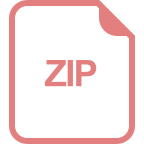














