用c语言实现给定两个链表,每个链表都已经按升序排列,设计算法实现将两个链表合并到一个升序链表中,返回合并后的链表。
时间: 2023-05-27 15:06:23 浏览: 124
```
#include <stdio.h>
#include <stdlib.h>
/* 链表结构体 */
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
/* 创建链表 */
ListNode* createList(int* nums, int numsSize) {
ListNode* head = NULL;
ListNode* tail = NULL;
for (int i = 0; i < numsSize; i++) {
ListNode* node = (ListNode*)malloc(sizeof(ListNode));
node->val = nums[i];
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = tail->next;
}
}
return head;
}
/* 合并两个链表 */
ListNode* mergeTwoLists(ListNode* l1, ListNode* l2) {
ListNode* head = NULL;
ListNode* tail = NULL;
while (l1 != NULL && l2 != NULL) {
ListNode* node = (ListNode*)malloc(sizeof(ListNode));
if (l1->val < l2->val) {
node->val = l1->val;
l1 = l1->next;
} else {
node->val = l2->val;
l2 = l2->next;
}
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = tail->next;
}
}
if (l1 != NULL) {
if (head == NULL) {
head = l1;
} else {
tail->next = l1;
}
}
if (l2 != NULL) {
if (head == NULL) {
head = l2;
} else {
tail->next = l2;
}
}
return head;
}
/* 输出链表 */
void printList(ListNode* head) {
while (head != NULL) {
printf("%d ", head->val);
head = head->next;
}
printf("\n");
}
int main() {
int nums1[] = {1, 2, 4};
int nums2[] = {1, 3, 4};
ListNode* l1 = createList(nums1, 3);
ListNode* l2 = createList(nums2, 3);
ListNode* merged = mergeTwoLists(l1, l2);
printList(merged);
return 0;
}
```
阅读全文
相关推荐
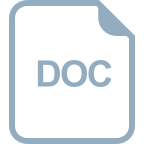
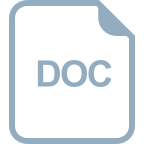
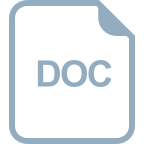

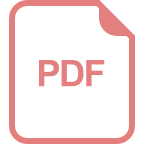
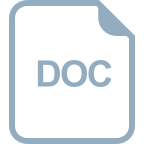
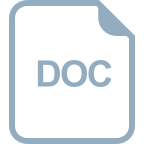
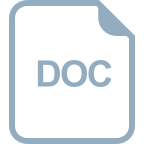
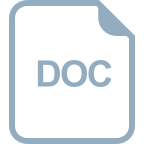






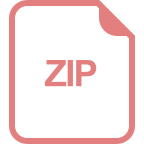