仅调用scanner一个库写RSA加密解密算法
时间: 2024-01-21 09:20:13 浏览: 80
RSA加密解密算法需要用到大数运算,Java中已经提供了BigInteger类用于处理大数运算,因此我们需要同时引入Scanner和BigInteger两个库。下面是一个简单的RSA加密解密代码示例:
```java
import java.util.Scanner;
import java.math.BigInteger;
public class RSA {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// 1. 选取两个不同的质数p和q
System.out.print("Enter a prime number p: ");
BigInteger p = sc.nextBigInteger();
System.out.print("Enter another prime number q: ");
BigInteger q = sc.nextBigInteger();
// 2. 计算n = p * q 和φ(n) = (p - 1) * (q - 1)
BigInteger n = p.multiply(q);
BigInteger phi_n = p.subtract(BigInteger.ONE).multiply(q.subtract(BigInteger.ONE));
// 3. 选择一个小于φ(n)的整数e,使得e与φ(n)互质
System.out.print("Choose a number e that is relatively prime to " + phi_n + ": ");
BigInteger e = sc.nextBigInteger();
// 4. 计算e关于φ(n)的模反元素d
BigInteger d = e.modInverse(phi_n);
// 输出公钥和私钥
System.out.println("Public key: (" + n + ", " + e + ")");
System.out.println("Private key: (" + n + ", " + d + ")");
// 加密
System.out.print("Enter a message to encrypt: ");
BigInteger message = sc.nextBigInteger();
BigInteger encrypted = message.modPow(e, n);
System.out.println("Encrypted message: " + encrypted);
// 解密
System.out.print("Enter a message to decrypt: ");
BigInteger cipher = sc.nextBigInteger();
BigInteger decrypted = cipher.modPow(d, n);
System.out.println("Decrypted message: " + decrypted);
}
}
```
注意,在输入p、q和e时,需要保证它们是质数或者与φ(n)互质的整数。此外,为了保证加密后的结果不超过n,输入的明文也需要是一个小于n的正整数。
阅读全文
相关推荐
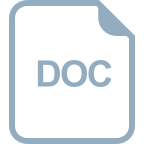
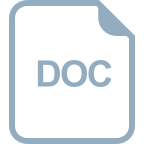
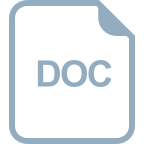

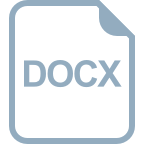
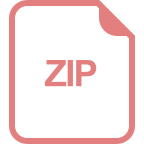
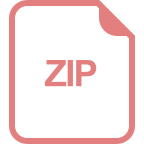
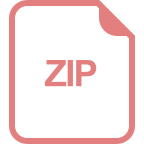
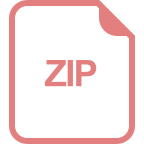
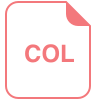
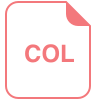
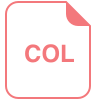
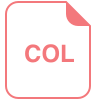
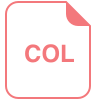
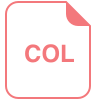
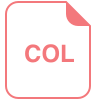
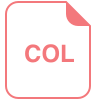
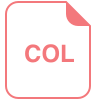
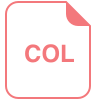