仅调用scanner一个库并且只需输入明文的写RSA加密解密算法
时间: 2024-01-21 09:20:14 浏览: 66
RSA加密解密算法需要使用Java的BigInteger类库来处理大数运算,下面是一个简单的示例代码:
```java
import java.math.BigInteger;
import java.util.Scanner;
public class RSADemo {
private static final BigInteger ONE = BigInteger.ONE;
private static final BigInteger ZERO = BigInteger.ZERO;
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入明文:");
String plaintext = scanner.nextLine();
// 生成RSA公私钥对
BigInteger[] keys = generateKeys(1024);
BigInteger n = keys[0];
BigInteger e = keys[1];
BigInteger d = keys[2];
// 显示公钥和私钥
System.out.println("公钥:(" + n + ", " + e + ")");
System.out.println("私钥:(" + n + ", " + d + ")");
// 加密明文
BigInteger plaintextNum = new BigInteger(plaintext.getBytes());
BigInteger ciphertextNum = encrypt(plaintextNum, e, n);
System.out.println("密文:" + ciphertextNum.toString());
// 解密密文
BigInteger decryptedNum = decrypt(ciphertextNum, d, n);
String decryptedText = new String(decryptedNum.toByteArray());
System.out.println("解密结果:" + decryptedText);
}
// 生成RSA公私钥对
private static BigInteger[] generateKeys(int keySize) {
BigInteger p = BigInteger.probablePrime(keySize / 2, new java.util.Random());
BigInteger q = BigInteger.probablePrime(keySize / 2, new java.util.Random());
BigInteger n = p.multiply(q);
BigInteger phi = p.subtract(ONE).multiply(q.subtract(ONE));
BigInteger e, d;
do {
e = new BigInteger(keySize, new java.util.Random());
} while (e.compareTo(phi) != -1 || !e.gcd(phi).equals(ONE));
d = e.modInverse(phi);
return new BigInteger[] { n, e, d };
}
// RSA加密
private static BigInteger encrypt(BigInteger plaintext, BigInteger e, BigInteger n) {
return plaintext.modPow(e, n);
}
// RSA解密
private static BigInteger decrypt(BigInteger ciphertext, BigInteger d, BigInteger n) {
return ciphertext.modPow(d, n);
}
}
```
该程序首先要求用户输入明文,然后生成一个大小为1024位的RSA公私钥对,并显示出来。接着使用公钥对明文进行加密,将密文输出到控制台。最后使用私钥对密文进行解密,并将解密结果输出到控制台。
阅读全文
相关推荐

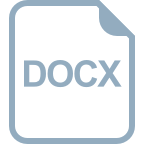
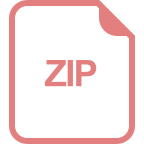
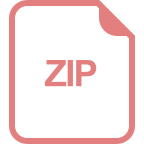
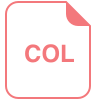
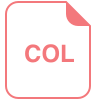
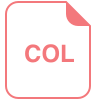
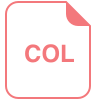
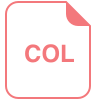
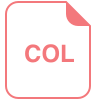
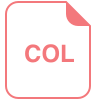
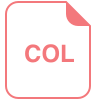