java实现rsa算法对文件加密解密,只使用biglnteger库与scanner库
时间: 2023-12-25 10:06:58 浏览: 166
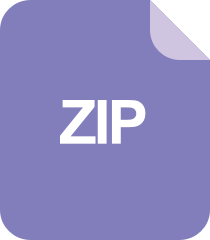
java,RSA算法加密文件

RSA算法是一种非对称加密算法,它涉及到大数运算,因此需要使用 BigInteger 库。下面是一个简单的Java实现RSA算法对文件进行加密和解密的示例代码,同时使用了 Scanner 库来读取用户输入的文件名和密钥:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.math.BigInteger;
import java.util.Scanner;
public class RSAEncryption {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 读取需要加密/解密的文件名
System.out.println("请输入需要加密/解密的文件名:");
String fileName = scanner.nextLine();
File file = new File(fileName);
// 读取密钥
System.out.println("请输入密钥:");
BigInteger privateKey = scanner.nextBigInteger();
BigInteger publicKey = scanner.nextBigInteger();
BigInteger modulus = scanner.nextBigInteger();
try {
if (file.exists()) {
FileInputStream fis = new FileInputStream(file);
byte[] data = new byte[(int) file.length()];
fis.read(data);
fis.close();
// 加密
byte[] encryptedData = encrypt(data, publicKey, modulus);
// 将加密后的数据写入新文件
FileOutputStream fos = new FileOutputStream(file.getName() + ".encrypted");
fos.write(encryptedData);
fos.close();
// 解密
byte[] decryptedData = decrypt(encryptedData, privateKey, modulus);
// 将解密后的数据写入新文件
FileOutputStream fos2 = new FileOutputStream(file.getName() + ".decrypted");
fos2.write(decryptedData);
fos2.close();
System.out.println("加密/解密完成!");
} else {
System.out.println("文件不存在!");
}
} catch (Exception e) {
e.printStackTrace();
}
}
// 加密
public static byte[] encrypt(byte[] data, BigInteger publicKey, BigInteger modulus) {
return new BigInteger(data).modPow(publicKey, modulus).toByteArray();
}
// 解密
public static byte[] decrypt(byte[] data, BigInteger privateKey, BigInteger modulus) {
return new BigInteger(data).modPow(privateKey, modulus).toByteArray();
}
}
```
需要注意的是,由于 RSA 算法涉及到大数运算,因此在加密/解密文件时可能会出现内存溢出等问题。如果需要处理大文件,建议使用流式加密/解密方式,而不是一次性将整个文件读入内存中进行处理。
阅读全文
相关推荐
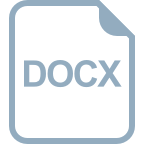
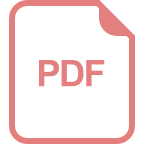
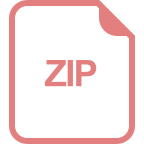
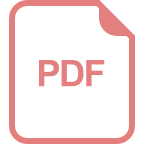
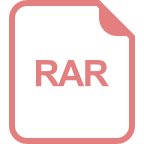
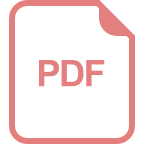
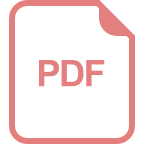
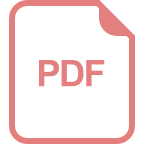
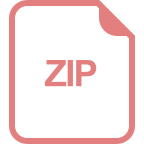
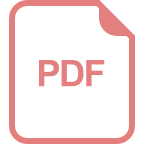
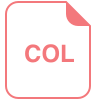
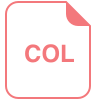
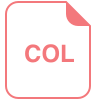
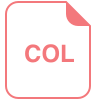

