python面向对象人机猜拳游戏
时间: 2023-06-28 16:07:39 浏览: 85
好的,下面是一个简单的 Python 面向对象人机猜拳游戏示例:
```python
import random
class Player:
def __init__(self, name):
self.name = name
self.score = 0
def choose_gesture(self):
pass
class HumanPlayer(Player):
def choose_gesture(self):
gesture = input(f"{self.name}, please choose your gesture (rock/paper/scissors): ")
while gesture not in ["rock", "paper", "scissors"]:
print("Invalid input. Please choose again.")
gesture = input(f"{self.name}, please choose your gesture (rock/paper/scissors): ")
return gesture
class ComputerPlayer(Player):
def choose_gesture(self):
return random.choice(["rock", "paper", "scissors"])
class Game:
def __init__(self, rounds):
self.rounds = rounds
self.human_player = HumanPlayer("Player 1")
self.computer_player = ComputerPlayer("Player 2")
def play_round(self):
human_gesture = self.human_player.choose_gesture()
computer_gesture = self.computer_player.choose_gesture()
if human_gesture == computer_gesture:
print("Tie!")
elif (human_gesture == "rock" and computer_gesture == "scissors") or \
(human_gesture == "paper" and computer_gesture == "rock") or \
(human_gesture == "scissors" and computer_gesture == "paper"):
print(f"{self.human_player.name} wins this round!")
self.human_player.score += 1
else:
print(f"{self.computer_player.name} wins this round!")
self.computer_player.score += 1
def play_game(self):
for i in range(self.rounds):
print(f"Round {i+1}:")
self.play_round()
print(f"Score: {self.human_player.name} {self.human_player.score} - {self.computer_player.score} {self.computer_player.name}")
if self.human_player.score > self.computer_player.score:
print(f"{self.human_player.name} wins the game!")
elif self.human_player.score < self.computer_player.score:
print(f"{self.computer_player.name} wins the game!")
else:
print("Tie game!")
if __name__ == "__main__":
game = Game(rounds=3)
game.play_game()
```
这个示例包含了 `Player` 和其子类 `HumanPlayer` 和 `ComputerPlayer`。`Player` 是一个基类,它定义了一个 `name` 属性和一个 `score` 属性,以及一个抽象方法 `choose_gesture`,该方法应该在子类中实现。`HumanPlayer` 和 `ComputerPlayer` 分别实现了 `choose_gesture` 方法,用于让人类玩家和计算机玩家进行选择手势。
`Game` 类定义了一个构造函数,该函数接受一些回合数,并创建了一个人类玩家和一个计算机玩家。`play_round` 方法进行了一轮游戏,让人类玩家和计算机玩家进行手势选择,并根据规则决定谁赢了这一轮。`play_game` 方法循环播放多个回合,并在最后输出获胜者。
在主程序中,我们创建了一个 `Game` 对象并调用了 `play_game` 方法进行游戏。
相关推荐














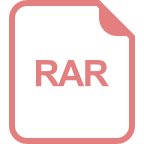
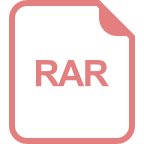