10T文件怎么用socketChannel从客户端传输到服务端 完整优化代码
时间: 2024-04-12 08:28:54 浏览: 147
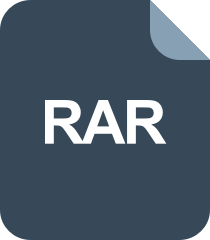
实现客户端与服务端的文件传输

下面是一个基于 Java NIO 的完整优化代码示例,用于将10TB文件使用 SocketChannel 从客户端传输到服务端:
客户端代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.channels.SocketChannel;
public class Client {
private static final int BUFFER_SIZE = 4096;
private static final String FILE_PATH = "path/to/10TB/file";
public static void main(String[] args) {
try {
// 打开文件通道
FileChannel fileChannel = new FileInputStream(new File(FILE_PATH)).getChannel();
// 创建SocketChannel并连接服务端
SocketChannel socketChannel = SocketChannel.open();
socketChannel.connect(new InetSocketAddress("server_ip", server_port));
// 循环读取文件数据并写入SocketChannel
ByteBuffer buffer = ByteBuffer.allocate(BUFFER_SIZE);
while (fileChannel.read(buffer) != -1) {
buffer.flip();
socketChannel.write(buffer);
buffer.clear();
}
// 关闭资源
fileChannel.close();
socketChannel.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
服务端代码:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
public class Server {
private static final int BUFFER_SIZE = 4096;
private static final String OUTPUT_FILE_PATH = "path/to/output/file";
public static void main(String[] args) {
try {
// 创建ServerSocketChannel并绑定到指定端口
ServerSocketChannel serverSocketChannel = ServerSocketChannel.open();
serverSocketChannel.bind(new InetSocketAddress(server_port));
// 等待客户端连接
SocketChannel socketChannel = serverSocketChannel.accept();
// 创建文件通道
FileChannel fileChannel = new FileOutputStream(OUTPUT_FILE_PATH).getChannel();
// 循环读取SocketChannel数据并写入文件通道
ByteBuffer buffer = ByteBuffer.allocate(BUFFER_SIZE);
while (socketChannel.read(buffer) != -1) {
buffer.flip();
fileChannel.write(buffer);
buffer.clear();
}
// 关闭资源
fileChannel.close();
socketChannel.close();
serverSocketChannel.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请注意替换代码中的 `server_ip`、`server_port`、`FILE_PATH` 和 `OUTPUT_FILE_PATH` 为实际的值。此示例仅作为基本框架,你可能需要根据实际需求进行进一步的错误处理和优化。
阅读全文
相关推荐


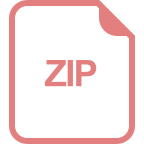





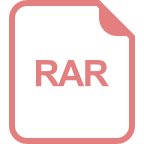
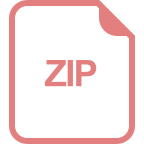





