java 使用Selector 实现SocketChannel传输和接收文件
时间: 2024-04-15 17:24:35 浏览: 106
要使用Selector实现SocketChannel的文件传输和接收,可以按照以下步骤进行操作:
1. 创建一个Selector对象,并将SocketChannel注册到该Selector上。可以使用`Selector.open()`方法创建Selector对象,然后使用`channel.register(selector, SelectionKey.OP_CONNECT)`将SocketChannel注册到Selector上,指定感兴趣的事件为连接操作。
2. 在循环中,使用Selector的`select()`方法等待就绪的事件。如果有就绪的事件,可以使用`selector.selectedKeys()`方法获取就绪的SelectionKey集合。
3. 遍历就绪的SelectionKey集合,处理每个就绪的事件。如果事件是连接操作(`SelectionKey.OP_CONNECT`),则完成连接并将SocketChannel注册为读操作。
4. 如果事件是读操作(`SelectionKey.OP_READ`),则从SocketChannel中读取数据并写入文件。可以使用`FileChannel.write()`方法将读取到的数据写入文件。
5. 检查是否已经将文件的所有数据接收完毕。可以使用FileChannel的`position()`方法获取当前文件的写入位置,如果写入位置等于文件大小,则表示所有数据已经接收完毕。
6. 关闭文件通道和SocketChannel,并释放资源。
下面是一个简单的示例代码:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.channels.Selector;
import java.nio.channels.SelectionKey;
import java.nio.channels.SocketChannel;
import java.util.Iterator;
public class FileTransferExample {
public static void main(String[] args) {
try {
Selector selector = Selector.open();
SocketChannel socketChannel = SocketChannel.open();
socketChannel.configureBlocking(false);
socketChannel.connect(new InetSocketAddress("localhost", 12345));
socketChannel.register(selector, SelectionKey.OP_CONNECT);
while (true) {
selector.select();
Iterator<SelectionKey> keyIterator = selector.selectedKeys().iterator();
while (keyIterator.hasNext()) {
SelectionKey key = keyIterator.next();
keyIterator.remove();
if (key.isConnectable()) {
SocketChannel channel = (SocketChannel) key.channel();
if (channel.isConnectionPending()) {
channel.finishConnect();
}
channel.register(selector, SelectionKey.OP_READ);
}
if (key.isReadable()) {
FileChannel fileChannel = new FileOutputStream("path/to/destination/file").getChannel();
ByteBuffer buffer = ByteBuffer.allocate(1024);
int bytesRead = socketChannel.read(buffer);
if (bytesRead == -1) {
fileChannel.close();
socketChannel.close();
System.out.println("File transfer completed.");
return;
}
buffer.flip();
fileChannel.write(buffer);
buffer.clear();
}
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请注意,这只是一个简单的示例代码,并没有处理所有的异常情况。在实际开发中,还需要考虑处理连接错误、读写错误等异常情况,并进行适当的错误处理。
阅读全文
相关推荐
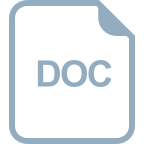
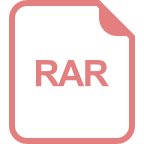
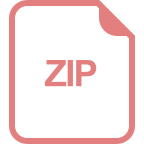
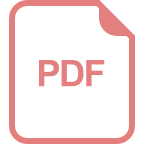
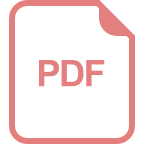
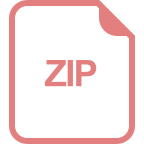
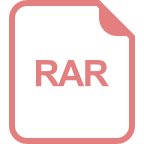
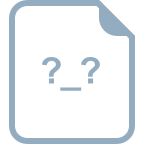
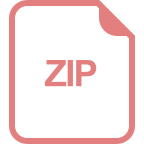
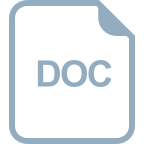
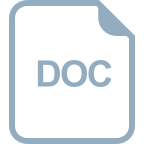
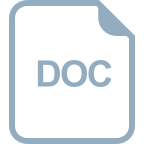
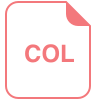
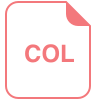
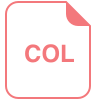