1)实现二叉树ADT的各种基本运算。 2)设计递归算法: ① 构建一棵二叉树。 ② 求一棵二叉树的高度。 ③ 求一棵二叉树中叶子结点数。 ④ 复制一棵二叉树。 ⑤ 交换一棵二叉树的左右子树。 3)设计main函数,测试上述每个运算
时间: 2024-10-31 11:06:51 浏览: 33
1) 实现二叉树 ADT 的各种基本运算通常包括:
- 插入节点 (Insert): 向树中添加新节点,保持二叉树性质(左子树小于根,右子树大于根)。
- 删除节点 (Delete): 根据给定值从树中移除节点,保持树的结构完整。
- 查找节点 (Search): 搜索特定值是否存在于树中。
- 遍历 (Traversal): 遍历所有节点,常见的有前序遍历 (根-左-右),中序遍历 (左-根-右) 和后序遍历 (左-右-根)。
- 获取大小 (Size): 计算树中节点的数量。
- 获取根 (Root): 返回树的根节点。
2) 设计递归算法:
① 构建一棵二叉树:
```java
void buildTree(Node root, int[] inorder, int start, int end) {
if (start > end)
return;
int mid = findMid(inorder, start, end);
root = new Node(inorder[mid]);
root.left = buildTree(root.left, inorder, start, mid - 1);
root.right = buildTree(root.right, inorder, mid + 1, end);
}
```
(其他递归方法同理)
② 求高度:
```java
int height(Node root) {
if (root == null)
return 0;
else
return Math.max(height(root.left), height(root.right)) + 1;
}
```
③ 求叶子节点数:
```java
int leafCount(Node root) {
if (root == null)
return 0;
else if (root.left == null && root.right == null)
return 1;
else
return leafCount(root.left) + leafCount(root.right);
}
```
④ 复制一棵二叉树:
```java
Node copyTree(Node root) {
if (root == null)
return null;
return new Node(root.val, copyTree(root.left), copyTree(root.right));
}
```
⑤ 交换左右子树:
```java
void swapLeftRight(Node &root) {
if (root != null) {
Node temp = root.left;
root.left = root.right;
root.right = temp;
}
}
```
3) 测试 main 函数示例:
```java
public static void main(String[] args) {
// 初始化树、数组等数据
Node tree = buildTree(null, ...); // 根据数据构造树
System.out.println("Height: " + height(tree));
System.out.println("Leaf Count: " + leafCount(tree));
Node copiedTree = copyTree(tree);
// 更改原树的某部分并观察影响
swapLeftRight(tree);
// 输出复制后的结果
}
```
记得替换 `buildTree`, `height`, `leafCount` 中的具体实现细节。
阅读全文
相关推荐
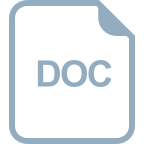
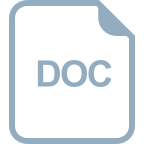
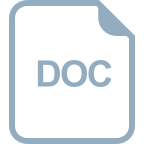
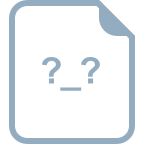
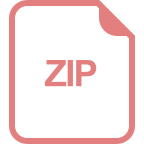
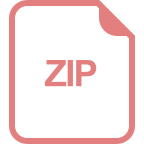
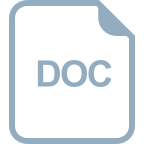
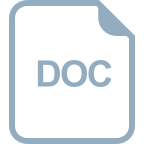
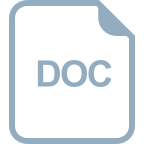
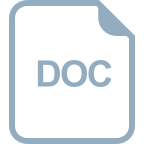
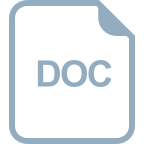
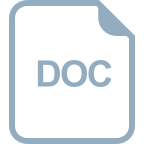
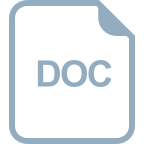
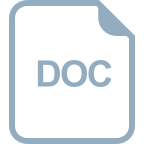
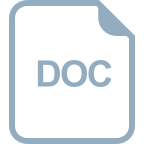



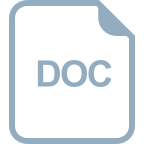