使用c++监视名为source.txt的文件,每当该文件内容发生变化时,它都会将更新后的文本追加到dest.txt
时间: 2024-09-30 08:12:57 浏览: 36
在C++中,你可以通过操作系统提供的功能,如`inotify`(Linux系统)或Windows的`ReadDirectoryChangesW`函数,来实现在源文件(source.txt)内容变化时自动监控并更新目标文件(dest.txt)。下面是一个简化的示例,展示了如何使用`boost::filesystem`库配合`inotify`(假设你的系统支持):
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <boost/filesystem.hpp>
// 如果你的系统不支持inotify,可以考虑其他方案,如定时检查文件更改
namespace fs = boost::filesystem;
void watch_file(const std::string& source_path, const std::string& dest_path) {
try {
// 创建一个目录描述符
int wd = inotify_init();
if (wd == -1) {
throw std::runtime_error("Failed to initialize inotify");
}
// 将source.txt添加到观察列表
struct INotifyEvent event;
do {
ssize_t ret = inotify_add_watch(wd, source_path.c_str(), IN_MODIFY);
if (ret == -1 && errno != ENOSPC) {
throw std::runtime_error("Failed to add watch for " + source_path);
}
} while (ret == -1);
// 检查并处理事件
while (true) {
ret = read(wd, &event, sizeof(event));
if (ret == -1) {
throw std::runtime_error("Error reading from inotify");
}
// 简单的示例:只处理修改事件
if (event.mask & IN_MODIFY) {
std::ifstream src(source_path, std::ios::binary);
std::ofstream dst(dest_path, std::ios::app | std::ios::binary);
dst << src.rdbuf(); // 更新dest.txt的内容
src.close();
dst.close();
// 清理INOTIFY事件,仅做演示
inotify_rm_watch(wd, event.wd);
}
}
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
}
int main() {
watch_file("source.txt", "dest.txt");
return 0;
}
```
阅读全文
相关推荐
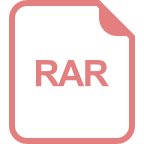
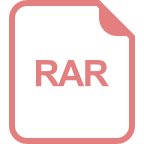
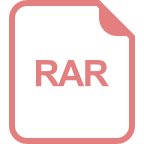
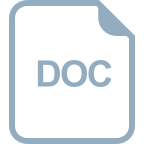
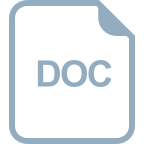
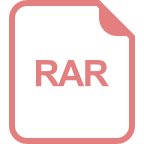
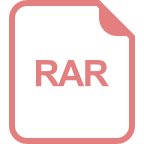
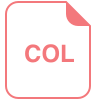
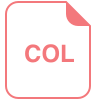
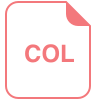
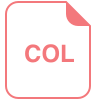
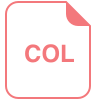
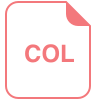



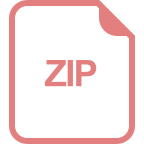
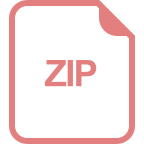
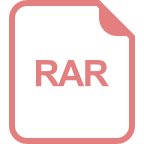