编写一个C程序来(i)使用整数数组实现堆栈的以下函数:stack_init():使堆栈为空为空():如果堆栈为空,则返回true。如果堆栈不是空的,则返回false(val):将项目val添加到堆栈弹出():删除最近添加到堆栈的顶部():返回最近添加到堆栈中的项目,但不要删除它。
时间: 2024-10-10 09:04:57 浏览: 18
为了编写一个C程序来实现上述堆栈功能,我们可以创建一个名为`stack.h`的头文件,其中包含堆栈结构体、函数声明以及相关的宏:
```c
// stack.h
#ifndef STACK_H
#define STACK_H
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int* items;
size_t capacity;
size_t top;
} Stack;
Stack* stack_init();
int stack_is_empty(Stack*);
void stack_push(Stack*, int);
int stack_pop(Stack*);
int stack_peek(Stack*);
#endif // STACK_H
```
然后,在`stack.c`文件中实现这些函数:
```c
// stack.c
#include "stack.h"
Stack* stack_init() {
Stack* s = (Stack*)malloc(sizeof(Stack));
s->items = NULL;
s->capacity = 0;
s->top = -1;
return s;
}
int stack_is_empty(Stack* s) {
return s->top == -1;
}
void stack_push(Stack* s, int val) {
if (s->top >= s->capacity - 1) {
s->capacity *= 2;
s->items = (int*)realloc(s->items, s->capacity * sizeof(int));
}
s->items[++s->top] = val;
}
int stack_pop(Stack* s) {
if (stack_is_empty(s)) {
printf("Error: Pop from empty stack.\n");
return -1;
}
return s->items[s->top--];
}
int stack_peek(Stack* s) {
if (stack_is_empty(s)) {
printf("Error: Peek on empty stack.\n");
return -1;
}
return s->items[s->top];
}
```
现在你可以使用`stack.h`文件中的函数来操作堆栈。例如:
```c
#include "stack.h"
int main() {
Stack* myStack = stack_init();
// 使用stack_push()添加元素
stack_push(myStack, 5);
stack_push(myStack, 10);
// 检查是否为空
if (!stack_is_empty(myStack)) {
printf("Stack is not empty.\n");
} else {
printf("Stack is empty.\n");
}
// 弹出并打印元素
int poppedVal = stack_pop(myStack);
printf("Popped value: %d\n", poppedVal);
// 查看顶部元素但不删除
int peekedVal = stack_peek(myStack);
printf("Peeked value: %d\n", peekedVal);
free(myStack);
return 0;
}
```
阅读全文
相关推荐
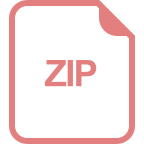
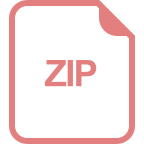
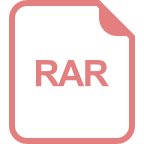















