图书管理系统代码C++
时间: 2024-06-13 07:01:42 浏览: 97
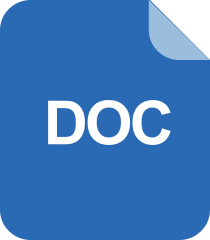
c++图书管理系统代码

图书管理系统是一个常见的管理系统,主要用于管理图书的借阅、归还、查询等操作。其实现涉及到数据结构和算法等多方面知识。下面是一个简单的C++代码示例:
```
#include <iostream>
#include <string>
using namespace std;
class Book {
public:
string name;
string author;
string press;
int stock;
int borrowed;
Book(string n, string a, string p, int s) {
name = n;
author = a;
press = p;
stock = s;
borrowed = 0;
}
};
class Library {
public:
Book books[100];
int numBooks;
Library() {
numBooks = 0;
}
void addBook(string n, string a, string p, int s) {
if (numBooks >= 100) {
cout << "The library is full." << endl;
return;
}
books[numBooks] = Book(n, a, p, s);
numBooks++;
}
void showBookList() {
for (int i = 0; i < numBooks; i++) {
cout << i + 1 << ". " << books[i].name << " by " << books[i].author
<< " published by " << books[i].press << ", stock: " << books[i].stock
<< ", borrowed: " << books[i].borrowed << endl;
}
}
void borrowBook(int index) {
if (index < 0 || index >= numBooks) {
cout << "Invalid index." << endl;
return;
}
if (books[index].stock <= 0) {
cout << "The book is out of stock." << endl;
return;
}
books[index].stock--;
books[index].borrowed++;
cout << "Borrow success." << endl;
}
void returnBook(int index) {
if (index < 0 || index >= numBooks) {
cout << "Invalid index." << endl;
return;
}
if (books[index].borrowed <= 0) {
cout << "The book is not borrowed." << endl;
return;
}
books[index].stock++;
books[index].borrowed--;
cout << "Return success." << endl;
}
};
int main() {
Library library;
library.addBook("C++ Primer", "Stanley B. Lippman", "Addison-Wesley Professional", 10);
library.addBook("Effective C++", "Scott Meyers", "Addison-Wesley Professional", 5);
library.addBook("Thinking in C++", "Bruce Eckel", "Prentice Hall PTR", 8);
library.showBookList();
library.borrowBook(1);
library.showBookList();
library.returnBook(1);
library.showBookList();
return 0;
}
```
上述代码实现了图书管理系统的基本功能:添加书籍、展示书籍列表、借阅书籍、归还书籍。具体实现细节可以根据需求进行调整。如果您有其他问题或需要进一步了解,可以随时问我哦!
阅读全文
相关推荐
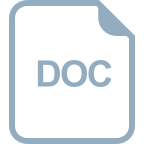
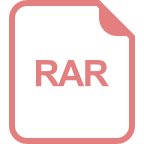
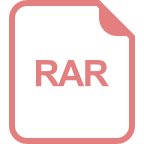
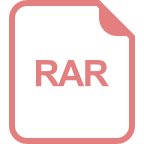
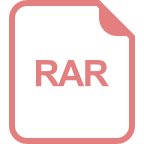
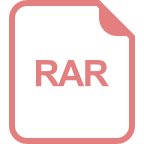
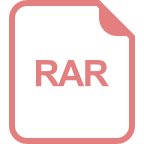
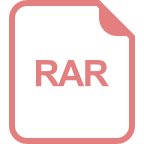
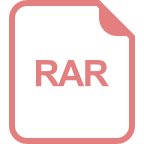
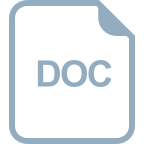
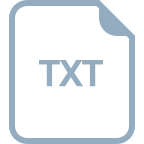
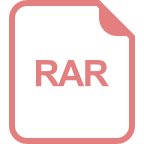
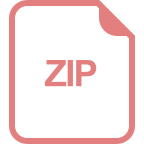
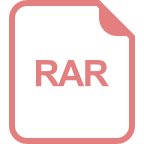